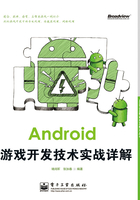
4.2 设置文本颜色
Color类的完整写法是Android.Graphics.Color,它的特点是设置颜色。虽然在Android平台上有很多种表示颜色的方法,但是类Color中提供的设置颜色方法是最好用的。在Color类中包含了如下12种最常用的颜色。
· Color.BLACK
· Color.BLUE
· Color.CYAN
· Color.DKGRAY
· Color.GRAY
· Color.GREEN
· Color.LTGRAY
· Color.MAGENTA
· Color.RED
· Color.TRANSPARENT
· Color.WHITE
· Color.YELLOW
类Color的3个静态方法,具体说明如下。
· static int argb(int alpha, int red, int green, int blue):构造一个包含透明对象的颜色;
· static int rgb(int red, int green, int blue):构造一个标准的颜色对象;
· static int parseColor(String colorString):解析一种颜色字符串的值,比如传入Color. BLACK。
Color类返回的都是整型结果,例如,返回0xff00ff00表示绿色、返回0xffff0000表示红色。我们可以将这个DWORD型看做AARRGGBB,AA代表Alpha透明色,后面的就不难理解了,每一段的范围为0~255。
实例4-1 用Color类更改文字的颜色(daima\4\yan)。
(1)设计理念
在本实例中,预先在Layout中插入了两个TextView控件,并通过两种程序的描述方法来实时更改原来Layout里TextView的背景色及文字颜色,最后使用类Android.Graphics.Color来更改文字的前景色。
(2)具体实现
step 1 编写布局文件main.xml,在里面使用了两个TextView对象,主要代码如下所示。
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" > <TextView android:id="@+id/myTextView01" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/str_textview01" /> <TextView android:id="@+id/myTextView02" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/str_textview02" /> </LinearLayout>
step 2 编写主文件yan.java,功能是调用各个公用文件来实现具体的功能。在里面分别新建了两个类成员变量mTextView01和mTextView02,这两个变量在onCreate之初,以findViewById方法使之初始化为Layout(main.xml)里的TextView对象。在当中使用了Resource类及Drawable类,分别创建了resources对象及HippoDrawable对象,调用了setBackgroundDrawable来更改mTextView01的文字底纹,并且使用setText方法来更改TextView里的文字。而在mTextView02中,使用了类Android.Graphics.Color中的颜色常数,并使用setTextColor来更改文字的前景色。文件yan.java的主要代码如下所示。
package dfzy.yan; import dfzy.yan.R; import android.app.Activity; import android.content.res.Resources; import android.graphics.Color; import android.graphics.drawable.Drawable; import android.os.Bundle; import android.widget.TextView; public class yan extends Activity { private TextView mTextView01; private TextView mTextView02; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); mTextView01 = (TextView) findViewById(R.id.myTextView01); mTextView01.setText("使用的是Drawable背景色文本。"); Resources resources = getBaseContext().getResources(); Drawable HippoDrawable = resources.getDrawable(R.drawable.white); mTextView01.setBackgroundDrawable(HippoDrawable); mTextView02 = (TextView) findViewById(R.id.myTextView02); mTextView02.setTextColor(Color.MAGENTA); } }
调试运行后的效果如图4-1所示。

图4-1 运行效果