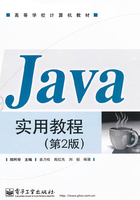
3.3 this引用
this是Java的关键字,用于表示对象自身的引用值。当在类中使用实例变量x或实例方法f()时,本质上都是this.x或this.f()。在不混淆的情况下(如没有名字隐藏),this.x可简写成x,this.f()可简写成f()。
当类中有两个同名变量,一个属于类的成员变量,另一个属于某个特定的方法(方法中的局部变量),可使用this区分成员变量和局部变量。使用this还可简化构造方法的调用。一个类的实例的成员方法在内存中只有一份备份,尽管在内存中可能有多个对象,而数据成员在类的每个对象所在内存中都存在一份备份。this引用允许相同的实例方法为不同的对象工作,每当调用一个实例方法时,引用该实例方法的特定类对象的this将自动传入实例方法代码中,方法的代码接着会与this所代表的对象的特定数据建立关联。在类的static方法中,是不能使用this的。这是因为类方法是一直存在,随时可用的,而此时可能该类一个对象都没有创建,自然this也就不存在。this通常用在构造方法实例变量初始化表达式、实例初始化代码块或实例方法中。除此之外出现this,编译报错。另外,认为this是当前对象的一个数据成员,这种理解是错误的。
【例3.7】 使用this引用来调用类A方法f1()。
TestThis.java
class A{ String name; public A(String str){ name = str; } public void f1(){ System.out.println("f1() of name "+ name+" is invoked!"); } public void f2(){ A a2 = new A("a2"); this.f1(); // 使用this引用调用f1()方法 a2.f1(); } } public class TestThis { public static void main(String[] args){ A a1 = new A("a1"); a1.f2(); } }
程序运行结果:
f1() of name a1 is invoked! f1() of name a2 is invoked!
可能在一个类中写了多个构造方法,若想在一个构造方法中调用另一个构造方法,以避免重复代码。可用this关键字来构成一个特殊的“显示构造方法”调用语句。该语句必须是构造方法体中第一条语句。格式为:this(参数列表);。
【例3.8】 使用this引用变量调用另一个构造方法。
ConWithThis.java
public class ConWithThis { int count = 0; String str = "hello"; ConWithThis(int i) { this("java"); // 调用ConWithThis(String s),必须是第一条语句 count = i; System.out.println("Constructor with int arg only, count= " + count); } ConWithThis(String s) { System.out.println("Constructor with String arg only, s = " + s); str = s; } ConWithThis(String s, int i) { this(i); // 调用ConWithThis(int i) this.str = s; System.out.println("Constructor with String and int args, s = " + s + ", i = " + i); } ConWithThis() { this("use this reference",9); // 调用ConWithThis(String s,int i),必须是第一条语句 System.out.println("default constructor(no args)"); } void f() { System.out.println("count = " + count + ", s = " + str); } public static void main(String[] args) { ConWithThis x = new ConWithThis(); x.f(); } }
程序运行结果:
Constructor with String arg only, s = java Constructor with int arg only, count= 9 Constructor with String and int args, s = use this reference, i = 9 default constructor(no args) count = 9, s = use this reference
说明:子类构造方法中第一条语句必须是super(…)或this(…),两者必居其一,而且只能有一条。不能有多条,否则报错。若没有明确调用super(…)或this(…),则编译程序自动插入super(…),成为第一条语句。