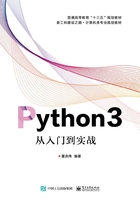
2.4 类型转换和输入
2.4.1 隐式类型转换
某些情况下,Python会自动将一个类型转为另外一个类型。例如,在进行整数和浮点数运算时,Python会自动将整数转为浮点数。同时,两个整数相除时也会自动转为浮点数的相除。例如:
a=25 b=3.14 c=a+b print("datatype of a:", type(a)) print("datatype of b:", type(b)) print("Value of c:", c) print("datatype of c:", type(c)) print(a/3)
输出:
datatype of a: <class 'int'> datatype of b: <class 'float'> Value of c: 28.14 datatype of c: <class 'float'> 8.333333333333334
但是,如果一个数(整数、浮点数等)和一个字符串想加,就不会进行“隐式类型转换”。例如:
print(30+"world")
将产生“不支持int和str的加法运算”的TypeError(类型错误):
---------------------------------------------------------------------- TypeError Traceback(most recent call last) <ipython-input-2-9869770bfa07> in <module>() ----> 1 print(30+"world") TypeError: unsupported operand type(s)for +: 'int' and 'str'
这时需要使用Python的内置函数进行“显式类型转换”。
2.4.2 显式类型转换
内置函数str()将数值类型(int、float、complex)的值转为字符串str类型。例如:
print(3*str(30)+"world") print(3*str(3.14)) print(3*str(3+6j))
输出:
303030world 3.143.143.14 (3+6j)(3+6j)(3+6j)
内置函数int()既可将一个合适格式的字符串类型的值转为int类型的值,也可以将一个float类型的值转为int类型的值(此时,该值小数点后的部分会被截断)。例如:
a=int(3.14) print(type(a)) print(a) a=int('1000') print(type(a)) print(a)
输出:
<class 'int'> 3 <class 'int'> 1000
但是,内置函数int()不能将一个不合适格式的字符串转为int类型的值。
a=int('1,000')
产生“无效的int文字量”的ValueError(值错误):
---------------------------------------------------------------------- ValueError Traceback(most recent call last) <ipython-input-3-4c775dde81c5> in <module>() ----> 1 a=int('1,000') ValueError: invalid literal for int()with base 10: '1,000'
内置函数float()既可将一个合适格式的字符串类型的值转为float类型的值,也可将一个int类型的值转为float类型的值。例如:
print(30+float('3.14')) print(float(314))
输出:
33.14 314.0
2.4.3 输入
可以通过内置函数input()从键盘输入数据,其语法格式为:
input(prompt='')
其中,prompt是一个用于提示信息的字符串。例如:
name=input("请输入你的用户名: ") print(name)
执行上述代码,输出结果如下:
请输入你的用户名:小白 小白
再如:
number=input("请输入一个数: ") print(type(number)) print(number+30)
第一个函数print()打印number的类型,第二个函数print()因int和str相加而产生TypeError(语法错误)。输出结果如下:
请输入一个数: 34 <class 'str'> ---------------------------------------------------------------------- TypeError Traceback(most recent call last) <ipython-input-6-9fef67d3d02c> in <module>() 1 number=input("请输入一个数: ") 2 print(type(number)) ----> 3 print(number+30) TypeError: must be str, not int
在执行number+30时出现了类型错误。函数input()输入的永远是一个字符串,所以让输入的字符串34和int类型的值30相加是非法的。正确的方法是将输入的代表数值的字符串用int()或float()函数转化为数值类型,再和int类型的值相加。
number=input("请输入一个数: ") print(float(number)+30)
执行:
请输入一个数: 34 64.0
总结
● 隐式类型转换:表达式中混合int、float类型的值时,Python会自动将int类型的值转为float类型的值。
● 显式类型转换:可以用内置函数str()将其他类型的值转为字符串str类型,也可以用内置函数int()或float()将其他类型的值转为int或float类型。
● 内置函数input()从键盘输入的永远是一个字符串str类型的值,需要用内置函数int()或float()转为数值类型才能参与算术计算。