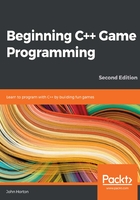
Arrays
If a variable is a box in which we can store a value of a specific type, such as int, float, or char, then we can think of an array as a row of boxes. The rows of boxes can be of almost any size and type, including objects made from classes. However, all the boxes must be of the same type.
Tip
The limitation of having to use the same type in each box can be circumvented to an extent once we learn some more advanced C++ in the penultimate project.
This array sounds like it could have been useful for our clouds in Chapter 2, Variables, Operators, and Decisions – Animating Sprites. So, how do we go about creating and using an array?
Declaring an array
We can declare an array of int type variables like this:
int someInts[10];
Now, we have an array called someInts that can store ten int values. Currently, however, it is empty.
Initializing the elements of an array
To add values to the elements of an array, we can use the type of syntax we are already familiar with while introducing some new syntax, known as array notation. In the following code, we store the value of 99 in the first element of the array:
someInts[0] = 99;
In order to store a value of 999 in the second element, we need to use the following code:
someInts[1] = 999;
We can store a value of 3 in the last element like this:
someInts[9] = 3;
Note that the elements of an array always start at zero and go upto the size of the array minus one. Similar to ordinary variables, we can manipulate the values stored in an array. The only difference is that we would use the array notation to do so because although our array has a name— someInts— the individual elements do not.
In the following code, we add the first and second elements together and store the answer in the third:
someInts[2] = someInts[0] + someInts[1];
Arrays can also interact seamlessly with regular variables, for example:
int a = 9999;
someInts[4] = a;
There are more ways we can initialize arrays, so let's look at one way now.
Quickly initializing the elements of an array
We can quickly add values to the elements as follows. This example uses a float array:
float myFloatingPointArray[3] {3.14f, 1.63f, 99.0f};
Now, the 3.14, 1.63, and 99.0 values are stored in the first, second, and third positions, respectively. Remember that, when using an array notation to access these values, we would use [0], [1], and [2].
There are other ways to initialize the elements of an array. This slightly abstract example shows using a for loop to put the values 0 through 9 into the uselessArray array:
for(int i = 0; i < 10; i++)
{
uselessArray[i] = i;
}
The preceding code assumes that uslessArray had previously been initialized to hold at least 10 int variables.
But why do we need arrays?
What do these arrays really do for our games?
We can use arrays anywhere a regular variable can be used – perhaps in an expression like this:
// someArray[4] is declared and initialized to 9999
for(int i = 0; i < someArray[4]; i++)
{
// Loop executes 9999 times
}
One of the biggest benefits of arrays in game code was hinted at at the start of this section. Arrays can hold objects (instances of classes). Let's imagine that we have a Zombie class and we want to store a whole bunch of them. We can do so like this:
Zombie horde [5] {zombie1, zombie2, zombie3}; // etc...
The horde array now holds a load of instances of the Zombie class. Each one is a separate, living (kind of), breathing, self-determining Zombie object. We could then loop through the horde array, each of which passes through the game loop, move the zombies, and check if their heads have met with an axe or if they have managed to catch the player.
Arrays, had we known about them at the time, would have been perfect for handling our clouds in Chapter 2, Variables, Operators, and Decisions – Animating Sprites. We could have had as many clouds as we wanted and written less code than we did for our three measly clouds.
Tip
To check out this improved cloud code in full and in action, look at the enhanced version of Timber!!! (code and playable game) in the download bundle. Alternatively, you can try to implement the clouds using arrays yourself before looking at the code.
The best way to get a feel for all of this array stuff is to see it in action. We will do this when we implement our tree branches.
For now, we will leave our cloud code as it is so that we can get back to adding features to the game as soon as possible. But first, let's do a bit more C++ decision-making with switch.