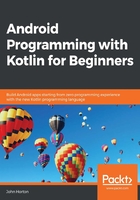
Laying out data with TableLayout
In the project window, expand the res
folder. Now, right-click the layout
folder and select New. Notice that there is an option for Layout resource file.
Select Layout resource file, and you will see the New Resource File dialog window.
In the File name field, enter my_table_layout
. This is the same name we used in the call to setContentView
within the loadTableLayout
function.
Notice that it has already selected LinearLayout as the Root element option. Delete LinearLayout
and type TableLayout
in its place.
Click the OK button and Android Studio will generate a new TableLayout
in an XML file called my_table_layout
and place it in the layout
folder ready for us to build our new table-based UI. Android Studio will also open the UI designer (if it isn't already) with the palette on the left and the attributes window on the right.
You can now uncomment the loadTableLayout
function:
fun loadTableLayout(v: View) { setContentView(R.layout.my_table_layout) }
You can now switch to the TableLayout
-based screen when you run the app, although currently, it is blank.
Adding a TableRow to TableLayout
Drag a TableRow
element from the Layouts
category on to the UI design. Notice that the appearance of this new TableRow
is virtually imperceptible, so much so that it is not worth inserting a diagram in the book. There is just a thin blue line at the top of the UI. This is because the TableRow
has collapsed itself around its content, which is currently empty.
It is possible to drag and drop our chosen UI elements onto this thin blue line, but it is also a little awkward, even counter intuitive. Furthermore, once we have multiple TableRow
elements next to each other, it gets even harder. The solution lies in the Component Tree window, which we introduced briefly when building the ConstraintLayout
.
Using the Component Tree when the visual designer won't do
Look at the Component Tree and notice how you can see the TableRow
as a child of the TableLayout
. We can drag our UI directly onto the TableRow
in the Component Tree. Drag three TextView
objects onto the TableRow
in the Component Tree and that should leave you with the following layout. I have photoshopped the following screenshot to show you the Component Tree and the regular UI designer in the same diagram:
Now add another two TableRow
objects (from the Layouts category). You can add them via the Component Tree window or the UI designer.
Tip
You need to drop them on the far-left of the window, otherwise the new TableRow
will become a child of the previous TableRow
. This will leave the whole table a bit of a muddle. If you accidentally add a TableRow
as a child of the previous TableRow,
you can either select it, then tap the Delete key, use the Ctrl + Z keyboard combination to undo it, or drag the mispositioned TableRow
to the left (in the Component Tree) to make it a child of the Table – as it should be.
Now, add three TextView
objects to each of the new TableRow
items. This will be most easily achieved by adding them via the Component Tree window. Check your layout to make sure it is as in the following screenshot:
Let's make the table look more like a genuine table of data that you might get in an app by changing some attributes.
On the TableLayout
, set the layout_width
and layout_height
attributes to wrap_content
. This gets rid of extra cells.
Change the color of all the outer (along the top and down the left-hand side) TextView
objects to black by editing the textColor
attribute. You achieve this by selecting the first TextView
, searching for its color
attribute, and then typing black
in the color
attribute values field. You will then be able to select @android:color/black
from a drop-down list. Do this for each of the outer TextView
elements.
Edit the padding
of each TextView
and change the all
attribute to 10sp
.
Organizing the table columns
It might seem at this point that we are done, but we need to organize the data better. Our table, like many tables, will have a blank cell in the top-left to divide the column and row titles. To achieve this, we need to number all the cells. For this, we need to edit the layout_column
attribute.
Tip
Cell numbers are numbered from zero from the left.
Start by deleting the top-left TextView
. Notice that the TextView
from the right has moved into the top-left position.
Next, in the new top-left TextView,
edit the layout_column
attribute to be 1
(this assigns it to the second cell, because the first is 0
and we want to leave the first one empty) and, for the next cell along, edit the layout_column
attribute to be 2
.
For the next two rows of cells, edit their layout_column
attributes from 0
to 2
from left to right.
If you want clarification on the precise code for this row after editing, here is a snippet, and remember to look in the download bundle in the Chapter04
/LayoutExploration
folder to see the whole file in context:
<TableRow android:layout_width="wrap_content" android:layout_height="wrap_content"> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_column="1" android:padding="10sp" android:text="India" android:textColor="@android:color/black" /> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_column="2" android:padding="10sp" android:text="England" android:textColor="@android:color/black" /> </TableRow>
Try to complete this exercise, however, using the Attributes window if possible.
Linking back to the main menu
Finally, for this layout, we will add a button that links back to the main menu. Add another TableRow
via the Component Tree. Drag a button onto the new TableRow
. Edit its layout_column
attribute to 1
so that it is in the middle of the row. Edit its text
attribute to Menu
and edit its onClick
attribute to match our already existing loadMenuLayout
function.
You can now run the app and switch back and forth between the different layouts.
If you want to, you can add some meaningful titles and data to the table by editing all the text
attributes of the TextView
widgets, as I have done in this following screenshot, showing the TableLayout
running in the emulator:
As a final thought, think about an app that presents tables of data. Chances are that data will be added to the table dynamically, not by the developer at design time as we have just done, but more likely by the user or from a database on the web. In Chapter 16, Adapters and Recyclers, we will see how to dynamically add data to different types of layout using adapters, and, in Chapter 27, Android Databases, we will also see how to create and use databases in our apps.