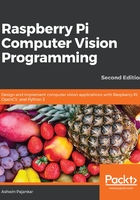
Understanding Python 3
Python is a high-level, interpreted, general-purpose programming language. It was created by Guido van Rossum and was started as a personal hobby project but has since grown into what it is today. The following is a timeline of the major milestones in the development of the Python programming language:

Figure 3.1 – Timeline of Python development milestones
Guido van Rossum held the title of benevolent dictator for life for the Python project for most of its life cycle. He stepped down from the role in July 2018 and has been part of the Python Steering Council ever since.
You can read more about Python on its home page at www.python.org.
The Python programming language has two major versions—Python 2 and Python 3. They are mostly incompatible with one another. As the preceding timeline shows, Python 2's sunset happened on 31st December 2019. This means that there is no further development of Python 2. Official support has also ceased to exist. The only Python version under active development and with continued support is Python 3. A lot of code (in fact, billions of lines of code) that is in production for many organizations is still in Python 2. So, the migration from Python 2 to Python 3 requires a major effort.
Python on RPi and Raspberry Pi OS
Python comes pre-installed on the Raspberry Pi OS image that we downloaded. Both versions of Python—Python 2 and Python 3—come with the Raspberry Pi OS image. We will look at Python 3 in detail as we will write all of our programs with Python 3.
Open lxterminal or log in remotely to the RPi and run the following command:
python -V
This produces the following output:
Python 2.7.16
The -V option returns the version of the Python interpreter. So, the python command refers to the Python 2 interpreter. However, we need Python 3. So, run the following command in the Command Prompt:
Python3 -V
This produces the following output:
Python 3.7.3
This is the Python 3 interpreter and we will use it for all of our programming exercises throughout this book. To find out the location of the interpreter on your disc (in our case, our microSD card), run the following command:
which python3
This produces the following output:
/usr/bin/python3
This is where the executable for the Python 3 interpreter is located.
Python 3 IDEs on Raspberry Pi OS
Before we get started with Python 3 programming, we will learn which Integrated Development Environments (IDEs) can be used to write programs with Python. Raspberry Pi OS, as of now, comes with two IDEs. Both can be accessed from the Programming option in the Raspbian menu, as shown:

Figure 3.2 – The Thonny and Geany Python IDEs in the Raspbian menu
The first option is the Geany IDE, which can be used with many programming and markup languages, including Python 2 and Python 3. You can read more about it at https://geany.org/. The second option is Thonny Python IDE, which supports Python 3 and the MicroPython variants.
I personally prefer to use Integrated Development and Learning Environment (IDLE), which is developed and maintained by the Python Foundation. You can read more about it at https://docs.python.org/3/library/idle.html. Earlier versions of Raspberry Pi OS used to come with IDLE. However, it is no longer present in the latest version of Raspberry Pi OS. Instead, we have Geany and Thonny. However, we can download IDLE with the following command:
sudo apt-get install idle3 -y
Once installed, we can find it in the Programming menu option under the Raspbian menu, as shown in the following screenshot:

Figure 3.3 – The option for IDLE for Python 3
Click on it to open it. Alternatively, we can launch it from the Command Prompt with the following command:
idle
Note that this command will not work and will throw an error if we have remotely connected to the Command Prompt of the RPi (using an SSH client such as PuTTY or Bitvise) as the command invokes the GUI. It will work if a visual display is directly connected to the RPi or if we access the RPi desktop remotely. This invokes a new window, as follows:

Figure 3.4 – Python 3 interactive mode in IDLE
This is the Python 3 interpreter prompt, or Python 3 shell. We will discuss this concept in detail later in this chapter.
Now, go to File | New File from the top menu. This will open a new code editor window, as follows:

Figure 3.5 – A new blank Python program
The interpreter window will also stay open when this happens. You can either close or minimize it. If you find it difficult to read the text in the interpreter or code editor in IDLE due to the size of the font, you can go to Options | Configure IDLE from the menu to set the font and size of the text. The configuration window looks as follows:

Figure 3.6 – IDLE configuration
Let's write a customary Hello World! program. Type the following text into the window:
print('Hello World!')
Then, from the menu, click Run | Run Module. It will ask you to save it. Click the OK button and it will take you to the Save dialog box. I prefer to save the code for this book by chapter in a directory, with sub-directories for each chapter. You can make the directory structure by running the following commands in the home directory of the pi user:
mkdir book
mkdir book/dataset
mkdir book/chapter01
We can make a separate directory for each chapter like this. Also, a separate dataset directory to store our data is needed. After creating the prescribed directory structure, run the following sequence of commands:
cd book
tree
We can see the directory structure in the following output of the tree command:

Figure 3.7 – Directory structure for saving programs for this book
We can create the same directory structure by using the Save dialog box of IDLE or the File Manager application of Raspberry Pi OS.
Once the directory corresponding to the current chapter is created, save the file there as prog00.py. You just need to enter the filename; IDLE will automatically assign the .py extension to the file. Then, the file will be executed by the Python 3 interpreter and the output will be visible in the interpreter shell, as follows:

Figure 3.8 – Execution of a Python 3 program in IDLE
We can also write the same code with the Nano editor. The only difference is that we also need to provide an extension when saving it. We can navigate to the directory that has the prog00.py file and run the following command to feed the file to the Python 3 interpreter:
python3 prog00.py
The Python 3 interpreter will execute the program and print the output, as follows:

Figure 3.9 – Execution of a Python 3 program in LXTerminal
Working with Python 3 in interactive mode
We have seen how to write a Python 3 program using the IDLE and Nano editors. We have also seen how to launch the program using IDLE and from the Command Prompt of Raspberry Pi OS. Running a Python 3 program in this fashion is known as script mode.
There is also another mode—interactive mode. In interactive mode, we launch the Python interpreter and it acts as a command-line interpreter. When we enter and run a statement, we get immediate feedback from the interpreter. We can launch the interactive mode in two ways. We have already seen the first way. When we launch IDLE, it opens the interpreter and we can use it to run the Python 3 statements. The other way is to run the python3 command in the Command Prompt. This will invoke the Python 3 interpreter in the Command Prompt, as follows:

Figure 3.10 – Python 3 in interactive mode on the Command Prompt
Type the following statement into the prompt:
>>> print('Hello World!')
Then, press Enter. It will be executed and the output will be shown on the next line. This way, we can execute single-line statements and small code snippets like this. We will be using interactive mode extensively in this chapter. From the next chapter onward, we will use script mode—that is, we will save the programs in files and launch them from the command prompt or IDLE.
The basics of Python 3 programming
Let's start by learning the basics of Python 3 programming. Open the Python 3 interactive prompt. Type in the following statements:
>>> pi = 3.14
>>> print(pi)
This will show the value of the pi variable. Run the following statement:
>>> print(type(3.14))
This shows the following output:
<class 'float'>
You might have noticed that we have not declared the data type of the variable here. That is because Python is a dynamically typed programming language. We also say that the variable belongs to a class type. This means that it is an object, which is true for all variables and other constructs in Python. Everything is an object in Python. This makes Python a truly object-oriented programming language. Almost everything has attributes and methods.
In order to exit the Command Prompt, press Ctrl + D or run the exit() statement.
Let's create our own class and an object of that class. Save the file and name it prog01.py, then add the following code to it:
class Person:
def __init__(self, name='', age=0):
self.name = name
self.age = age
def show(self):
print(self.name)
print(self.age)
In the preceding code, we defined Person. The __init__() class is the initializer function and it is called automatically whenever an object of the Person class is created. The self parameter is a reference to the current instance of the class and is used to access variables that belong to the class within the class definition.
Let's add some more code to prog01.py. We will create a class object, as follows:
p1 = Person('Ashwin', 25)
p1.show()
We created the p1 class and then showed the properties of the object with the show() function call. Here, we are assigning the values to class member variables at the time of the creation of the class object.
Let's look at another way to create an object and assign values to the member variables. Add the following code to the file:
p2 = Person()
p2.name = 'Jane'
p2.age = 20
print(p2.name)
print(p2.age)
In the preceding code, we are creating an object and the initializer function is called with the default arguments. Then, we are assigning the values to the class variables and accessing them directly using the class object. Run the program and see the output.
Now, open the Python 3 interpreter and run the following statements:
>>> import sys
>>> print(sys.platform)
This will return the name of the current OS (Linux). The first statement imports sys, which is a Python standard library. It comes with the Python interpreter as part of its batteries included motto. This means the Python interpreter comes with a large set of useful libraries. sys.platform returns the current OS name string.
Let's try another example. In the previous chapter, we installed the OpenCV library. Let's import that again now. We have already tested it directly from the Raspberry Pi OS Command Prompt. Let's try to do the same in interactive mode:
>>> import cv2
>>> print(cv2.__version__)
The first statement imports the OpenCV library to the current session. The second statement returns a string that contains the version number of the installed OpenCV library.
There are many topics in the basics of Python 3 programming, but it is very difficult to cover all of them. Also, to do so is beyond the scope of this book. However, we will use the topics we have just learned quite frequently in this book.
In the next section, we will explore the SciPy ecosystem libraries.