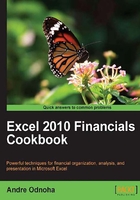
Scripting an Exchange server Installation
If you are performing mass deployment of Exchange servers in a large environment, automating the installation process can minimize administrator error and speed up the overall process. The setup.com
utility can be used to perform an unattended installation of Exchange, and, when combined with PowerShell and just a little bit of scripting logic, create a fairly sophisticated installation script. This recipe will provide a couple of examples that can be used to script the installation of an Exchange server.
Getting ready
You can use a standard PowerShell console from the server to run the scripts in this recipe.
How to do it...
- In this example, we'll create an automated installation script that installs Exchange based on the host name of the server. Using Notepad or your favourite scripting editor, add the following code to a new file:
if(Test-Path $Path) { switch -wildcard ($env:computername) { "*-HCM-*" {$role = "HT,CA,MB" ; break} "*-MB-*" {$role = "MB" ; break} "*-CA-*" {$role = "CA" ; break} "*-HT-*" {$role = "HT" ; break} "*-ET-*" {$role = "ET" ; break} "*-UM-*" {$role = "UM" ; break} } $setup = Join-Path $Path "setup.com" Invoke-Expression "$setup /InstallWindowsComponents /r:$role" } else { Write-Host "Invalid Media Path!" }
- Save the file as
InstallExchange.ps1
. - Execute the script from a server where you want to install Exchange using the following syntax:
InstallExchange.ps1 -Path D:
The value provided for the -Path
parameter should reference the Exchange 2010 SP1 media, either on DVD or extracted to a folder.
How it works...
One of the most common methods for automating an Exchange installation is determining the required roles based on the hostname of the server. In the previous example, we assume that your organization uses a standard server naming convention. When executing the script, the switch statement will evaluate the hostname of the server and determine the required roles. For example, if your mailbox servers use a server name such as CONTOSO-MB-01
, the mailbox server role will be installed. If your CAS servers use a server name such as CONTOSO-CA-02
, the CAS role will be installed, and so on.
It's important to note that Exchange 2010 SP1 requires several Windows operating system hotfixes. Windows Server 2008 R2 SP1 includes these operating system hotfixes required by Exchange 2010 SP1. You'll also want the .NET Framework 3.5.1 installed prior to running this script, which can also be automated using the ServerManager
PowerShell module that is included in Windows Server 2008 R2.
When calling the Setup.com
installation program within the script, we use the /InstallWindowsComponents
switch, which is a new Setup.com
feature in Exchange Server 2010 SP1. This will allow the setup program to load any prerequisite Windows roles and features, such as IIS, and so on, before starting the Exchange installation.
There's more...
Scripting the installation of Exchange based on the server names may not be an option for you. Fortunately, PowerShell gives us plenty of flexibility. The following script uses similar logic, but performs the installation based on different criteria.
Let's say that your core Exchange infrastructure has already been deployed. Your corporate headquarters already has the required CAS and Hub Transport server infrastructure in place and therefore you only need to deploy mailbox servers in the main Active Directory site. All remaining remote sites will contain multi-role Exchange servers. Replace the code in the InstallExchange.ps1
script with the following:
param($Path) $site = [DirectoryServices.ActiveDirectory.ActiveDirectorySite] if(Test-Path $Path) { switch ($site::GetComputerSite().Name) { "Headquarters" {$role = "MB"} Default {$role = "HT,CA,MB"} } $setup = Join-Path $Path "setup.com" Invoke-Expression "$setup /InstallWindowsComponents /r:$role" } else { Write-Host "Invalid Media Path!" }
This alternate version of the script determines the current Active Directory site of the computer executing the script. If the computer is in the Headquarters site, only the Mailbox role is installed. If it is located at any of the other remaining Active Directory sites, the Hub Transport, Client Access, and Mailbox server roles are installed.
As you can see, combining the Setup.com
utility with a PowerShell script can give you many more options when performing an automated installation.