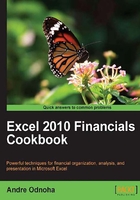
Creating recipients in bulk using a CSV file
One of the most common bulk provisioning techniques used in the Exchange Management Shell makes use of comma-separated value (CSV) files. These files act sort of like a database table. Each record in this table is represented by one line in the file, and each field value is separated by a comma, which is used as a delimiter. In this recipe, you'll learn how to set up a CSV file and create recipients in bulk using the Exchange Management Shell.
Getting ready
In addition to the Exchange Management Shell, you'll need to use Microsoft Excel to create a CSV file.
How to do it...
- In this example, we are going to create some mailboxes in bulk. We'll enter some data into Excel that will include the settings for five new mailboxes:
- Go to File | Save As and select CSV (Comma delimited) (*.csv) for the file type. Save the file as
C:\Mailboxes.CSV
. - Within the Exchange Management Shell, create a secure password object to be used as an initial password for each mailbox:
$pass = ConvertTo-SecureString -AsPlainText P@ssw0rd01 -Force
- Import the CSV file and create the mailboxes:
Import-CSV C:\Mailboxes.CSV | % { New-Mailbox -Name $_.Name ` -Alias $_.Alias ` -UserPrincipalName $_.UserPrincipalName ` -OrganizationalUnit $_.OrganizationalUnit ` -Password $pass ` -ResetPasswordOnNextLogon $true }
How it works...
In this example, we're importing the CSV file into the shell and piping that information to the ForEach-Object
cmdlet (using the %
alias). For each record in the CSV file, we're running the New-Mailbox
cmdlet, providing values for the -Name
, -Alias
, –UserPrincipalName,
and -OrganizationalUnit
parameters. The properties for each record can be accessed inside the loop using the $_
variable, which is the automatic variable that references the current object in the pipeline. The property names for each record match the header names used in the CSV file. As we create each mailbox, the password is set to the $pass
variable. The –ResetPasswordOnNextLogon
parameter is set to $true,
which will require each user to reset their password after their first logon.
Using this technique, you can literally create thousands of mailboxes in a matter of minutes. This concept can also be applied to other recipient types, such as distribution groups and contacts. You just need to specify the appropriate parameter values in the CSV file and use the corresponding cmdlet for the recipient type. For example, if you want to bulk provision contacts from a CSV file, use the code from the previous example as a guide, and, instead of using the New-Mailbox
cmdlet, use the New-MailContact
cmdlet and whatever parameters are required based on your settings.
There's more...
Let's take a look at an alternative approach to the previous example. Let's say that you don't want to set an initial password for each user, and, instead, you want to include this information in the CSV file so each new mailbox gets a unique password. Again, you'll need to set up a CSV file with the required values. For this example, your CSV file would look something like this:

Notice that in the previous screenshot, we are using different column names for this new file. We've removed the OrganizationalUnit
column and now have a Password
column which will be used to create each mailbox with a unique password. After you're done creating the file, save it again as C:\Mailboxes.CSV
.
Next, you can use the following code to create the mailboxes, specifying the path and file name to the CSV file created in the previous step:
Import-CSV C:\Mailboxes.CSV | % { $pass = ConvertTo-SecureString -AsPlainText $_.Password -Force New-Mailbox -Name $_.Name ` -Alias $_.Alias ` -UserPrincipalName $_.UserPrincipalName ` -Password $pass }
As we loop through each record in the CSV file, we create a secure password object that can be used with the -Password
parameter. The main difference here compared to the previous example is that each user gets a unique password and they do not need to reset their password the first time they log on.
Taking it a step further
When provisioning recipients you'll probably need to do multiple things, such as set Active Directory attributes and configure distribution group membership. Let's take our previous example a step further:
Import-CSV C:\NewMailboxes.CSV | % { New-Mailbox -Name $_.Name ` -FirstName $_.FirstName ` -LastName $_.LastName ` -Alias $_.Alias ` -UserPrincipalName $_.UserPrincipalName ` -Password $pass -OrganizationalUnit $_.OrganiationalUnit ` -Database DB1 ` -Password (ConvertTo-SecureString -AsPlainText P@ssw0rd -Force) Set-User -Identity $_.Name ` -City $_.City ` -StateOrProvince $_.State ` -Title $_.Title ` -Department $_.Department Add-DistributionGroupMember -Identity DL_Sales ` -Member $_.Name Add-DistributionGroupMember -Identity DL_Marketing ` -Member $_.Name }
Here we're still using a CSV file, but as we loop through each record we're calling multiple cmdlets to first create the mailbox, set some of the Active Directory attributes, and then add the mailbox to two separate distribution groups. In order to use this code, we would just need to create a CSV file that has columns for all of the values we're setting.
Now that we have this framework in place, we can add as many columns as we need to the CSV file and we can call any number of cmdlets for each record in the CSV.
See also
- Looping through items in Chapter 1, PowerShell Key Concepts
- Adding, modifying, and removing mailboxes
- Managing distribution groups