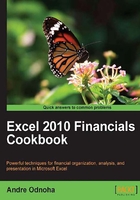
Adding and removing recipient e-mail addresses
There are several recipient types in Exchange 2010 and each one of them can support multiple e-mail addresses. Of course, the typical user mailbox recipient type is probably the first that comes to mind, but we also have distribution groups, contacts, and public folders, each of which can have one or more e-mail addresses. The syntax used for adding and removing e-mail addresses to each of these recipient types is essentially identical: the only thing that changes is the cmdlet that is used to set the address. In this recipe, you'll learn how to add or remove an e-mail address from an Exchange recipient.
How to do it...
- To add a secondary e-mail address to a mailbox, use the following command syntax:
Set-Mailbox dave -EmailAddresses @{add='dave@west.contoso.com'}
- Multiple addresses can also be added using this technique:
Set-Mailbox dave -EmailAddresses @{ add='dave@east.contoso.com', 'dave@west.contoso.com', 'dave@corp.contoso.com' }
- E-mail addresses can also be removed using the following syntax:
Set-Mailbox dave -EmailAddresses @{remove='dave@west.contoso.com'}
- Just as we are able to add multiple e-mail addresses at once, we can do the same when removing an address:
Set-Mailbox dave -EmailAddresses @{ remove='dave@east.contoso.com', 'dave@corp.contoso.com' }
How it works...
Adding and removing e-mail addresses was more challenging in the Exchange 2007 management shell because it required that you work directly with the EmailAddresses
collection, which is a multi-valued property. In order to modify the collection, you first had to save the object to a variable, modify it, and then write it back to the EmailAddresses
object on the recipient. This made it impossible to update the e-mail addresses for a recipient with one command.
The Set-*
cmdlets used to manage recipients in Exchange 2010 now support a new syntax that allows us to use a hash table to modify the EmailAddresses
property. As you can see from the code samples, we can simply use the Add
and Remove
keys within the hash table, and the assigned e-mail address values will be either added or removed as required. This is a nice change that makes it easier to do this in scripts and especially when working interactively in the shell.
The Add
and Remove
keywords are interchangeable with the plus (+
) and minus (-
) characters that serve as aliases:
Set-Mailbox dave -EmailAddresses @{ '+'='dave@east.contoso.com' '-'='dave@west.contoso.com' }
In the previous example, we've added and removed e-mail addresses from the mailbox. Notice that the +
and -
keywords need to be enclosed in quotes so PowerShell does not try to interpret them as the +=
and -=
operators.
This syntax works with all of the Set-*
cmdlets that support the -EmailAddresses
parameter:
Set-CASMailbox
Set-DistributionGroup
Set-DynamicDistributionGroup
Set-Mailbox
Set-MailContact
Set-MailPublicFolder
Set-MailUser
Keep in mind that the best way to add an e-mail address to a recipient is through the use of an e-mail address policy. This may not always be an option, but should be used first if you find yourself in a situation where addresses need to be added to a large number of recipients. With that said, it is possible do this in bulk using a simple foreach
loop:
foreach($i in Get-Mailbox -OrganizationalUnit Sales) { Set-Mailbox $i -EmailAddresses @{ add="$($i.alias)@west.contoso.com" } }
This code simply iterates over each mailbox in the Sales OU and adds a secondary e-mail address using the existing alias at west.contoso.com
. You can use this technique and modify the syntax as needed to perform bulk operations.
There's more...
Imagine a situation where you need to remove all e-mail addresses under a certain domain from all of your mailboxes. These could be secondary addresses that were added manually to each mailbox, or that used to be applied as part of an e-mail address policy that no longer applies. The following code can be used to remove all e-mail addresses from mailboxes under a specific domain:
foreach($i in Get-Mailbox -ResultSize Unlimited) { $i.EmailAddresses | ?{$_.SmtpAddress -like '*@corp.contoso.com'} | %{ Set-Mailbox $i -EmailAddresses @{remove=$_} } }
This code iterates through each mailbox in the organization and simply uses a filter to discover any e-mail addresses at corp.contoso.com
. If any exist, the Set-Mailbox
cmdlet will attempt to remove each of them from the mailbox.
See also
- Adding, modifying, and removing mailboxes
- Working with contacts
- Managing distribution groups