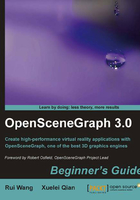
Time for action—reading the model filename from the command line
The most common public method of osg::ArgumentParser
is the overloaded read()
function. In this example, we are going to read command-line arguments with a special format and apply the parsing result to the osgDB::readNodeFile()
function.
- Include the necessary headers:
#include <osgDB/ReadFile> #include <osgViewer/Viewer>
- In the main function, try reading
--model
and thefilename
from the input arguments:osg::ArgumentParser arguments( &argc, argv ); std::string filename; arguments.read( "--model", filename );
- Read
Node
from the specified file and initialize the viewer. This is very similar to some previous examples except that it replaces theconst
string"Cessna.osg
" with astd::string
variable:osg::ref_ptr<osg::Node> root = osgDB::readNodeFile( filename ); osgViewer::Viewer viewer; viewer.setSceneData( root.get() ); return viewer.run();
- Build and start this example! Assuming that your executable file is
MyProject.exe
, type the following command in the prompt:# MyProject.exe --model dumptruck.osg
- We will see more than a Cessna model now. It is a dump truck loaded from the disk! Please be aware that you should have the OSG sample data installed, and the environment variable
OSG_FILE_PATH
set.
What just happened?
The dump truck model is loaded and rendered on the screen. Here, the most important point is that the filename dumptruck.osg
is obtained from the command-line argument. The read()
function, which consists of a format string parameter and a result parameter, helps to successfully find the first occurrence of the user-defined option --model
and the filename argument that follows.
The read()
function of the osg::ArgumentParser
class is overloaded. You may obtain integers, float and double values, and even mathematical vectors, in addition to strings, from its parameters. For instance, to read a customized option --size
with a single precision value from the command line, just use the following code:
float size = 0.0f; arguments.read( "--size", size );
The initial value of size
will not be changed if there is no such argument, --size
.