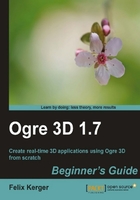
We will create a point light and add it to our scene to see the effect it has on our scene:
- Add the following code after setting the material for the plane:
Ogre::SceneNode* node = mSceneMgr->createSceneNode("Node1"); mSceneMgr->getRootSceneNode()->addChild(node);
- Create a light with the name
Light1
and tell Ogre 3D it's a point light:Ogre::Light* light1 = mSceneMgr->createLight("Light1"); light1->setType(Ogre::Light::LT_POINT);
- Set the light color and position:
light1->setPosition(0,20,0); light1->setDiffuseColour(1.0f,1.0f,1.0f);
- Create a sphere and set it at the position of the light, so we can see where the light is:
Ogre::Entity* LightEnt = mSceneMgr->createEntity("MyEntity","sphere.mesh"); Ogre::SceneNode* node3 = node->createChildSceneNode("node3"); node3->setScale(0.1f,0.1f,0.1f); node3->setPosition(0,20,0); node3->attachObject(LightEnt);
- Compile and run the application; you should see the stone texture lit by a white light, and see a white sphere a bit above the plane.
We added a point light to our scene and used a white sphere to mark the position of the light.
In step 1, we created a scene node and added it to the root scene node. We created the scene node because we need it later to attach the light sphere to. The first interesting thing happened in step 2. There we created a new light using the scene manager. Each light will need a unique name, if we decide to give it a name. If we decide not to use a name, then Ogre 3D will generate one for us. We used Light1
as a name. After creation, we told Ogre 3D that we want to create a point light. There are three different kinds of lights we can create, namely, point lights, spotlights, and directional lights. Here we created a point light; soon we will create the other types of lights. A point light can be thought of as being like a light bulb. It's a point in space which illuminates everything around it. In step 3, we used the created light and set the position of the light and its color. Every light color is described by a tuple (r,g,b). All three parameters have a range from 0.0 to 1.0 and represent the attribution of their assigned color part to the color. 'r' stands for red, 'g' for green, and 'b' for blue. (1.0,1.0,1.0) is white, (1.0,0.0,0.0) is red, and so on. The function we called was setDiffuseColour(r,g,b)
, which takes exactly these three parameters for the color. Step 4 added a white sphere at the position of the light, so we could see where the light is positioned in the scene.