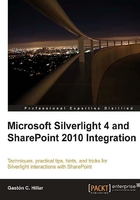
Creating a Silverlight LOB (Line-of-Business) RIA
Now, we are going to create a very simple Silverlight LOB (Line-Of-Business) RIA that retrieves data, displays a grid with a list of projects, and allows the users to navigate through the data. Then, we are going to integrate this Silverlight UI in SharePoint.
- Create a new Visual C# project using the Silverlight | Silverlight Application template. Use
SilverlightProjects
as the project's name. - Deactivate the Host the Silverlight application in a new Web site checkbox in the New Silverlight Application dialog box. We want the Silverlight application to run in a simple HTML web page. As you have installed Silverlight 4 Tools, the dialog box will offer you a combo box with the possibility to choose the desired Silverlight version. Select Silverlight 4 as we want to take advantage of the new features offered by this version.
- Add a new XML file to the project,
Projects.xml
. The following lines define properties and values for fiveproject
instances. This way, we have some data in XML format for our simple LOB application.<?xml version="1.0" encoding="utf-8" ?> <projects> <project projectId="0"> <title>Creating a Silverlight 4 UI</title> <estimatedDaysLeft>4</estimatedDaysLeft> <status>Delayed</status> <assignedTo>Jon Share</assignedTo> <numberOfTasks>5</numberOfTasks> </project> <project projectId="1"> <title>Creating a Complex Silverlight LOB RIA</title> <estimatedDaysLeft>5</estimatedDaysLeft> <status>Delayed</status> <assignedTo>James Point</assignedTo> <numberOfTasks>35</numberOfTasks> </project> <project projectId="2"> <title>Creating a New SharePoint Site</title> <estimatedDaysLeft>3</estimatedDaysLeft> <status>Delayed</status> <assignedTo>Vanessa Dotcom</assignedTo> <numberOfTasks>8</numberOfTasks> </project> <project projectId="3"> <title>Installing a New SharePoint 2010 Server</title> <estimatedDaysLeft>3</estimatedDaysLeft> <status>Delayed</status> <assignedTo>Michael Desktop</assignedTo> <numberOfTasks>25</numberOfTasks> </project> <project projectId="4"> <title>Testing the New Silverlight LOB RIA</title> <estimatedDaysLeft>4</estimatedDaysLeft> <status>Delayed</status> <assignedTo>Jon Share</assignedTo> <numberOfTasks>35</numberOfTasks> </project> </projects>
- Add a new class to the project called
Project
in a new class file,Project.cs
. The following lines define the new class, with six properties. This way, you will be able to create instances of this class to hold the values defined in the previously created XML file.public class Project { public int ProjectId { get; set; } public string Title { get; set; } public int EstimatedDaysLeft { get; set; } public string Status { get; set; } public string AssignedTo { get; set; } public int NumberOfTasks { get; set; } }
- Open
MainPage.xaml
, define a new width and height for theGrid
as800
and600
, add the following controls located in the Toolbox under All Silverlight Controls, and align them as shown in the screenshot. Remember that Visual Studio 2010 allows us to drag-and-drop controls from the toolbox to the SilverlightUserControl
in the design view and it will automatically generate the XAML code.- Two
Label
controls. - One
DataGrid
control and set its name todataGridProjects
. Set itsAutoGenerateColumns
property totrue.
- One
Slider
control,sliGridFontSize
. Set itsMinimum
property to8, Maximum
to72
, andValue
to11.
- Two
- Apply data binding to the font size for the
DataGrid
control,dataGridProjects
. In order to do so, selectdataGridProjects
, activate the Properties panel, display them in alphabetical order, right-click on the FontSize property, and select Apply Data Binding in the context menu that appears. Then, selectElementName
in Source,sliGridFontSize
, and thenValue
in Path. This way, when the user moves the slider, the font size for the data grid will change. The code that defines the data binding is as follows:FontSize="{Binding ElementName=sliGridFontSize, Path=Value}"
- The complete XAML markup code for
MainPage.xaml
will be similar to the following lines:<UserControl x:Class="SilverlightProjects.MainPage" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/ presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup- compatibility/2006" mc:Ignorable="d" d:DesignWidth="800" d:DesignHeight="600" xmlns:data= "clr-namespace:System.Windows.Controls; assembly=System.Windows.Controls.Data" xmlns:dataInput= "clr-namespace:System.Windows.Controls; assembly=System.Windows.Controls.Data.Input"> <Grid x:Name="LayoutRoot" Background="White"> <data:DataGrid AutoGenerateColumns="True" Height="491" HorizontalAlignment="Left" Margin="12,56,0,0" Name="dataGridProjects" VerticalAlignment="Top" Width="776" FontSize="{ Binding ElementName=sliGridFontSize, Path=Value}" /> <dataInput:Label Height="38" HorizontalAlignment="Left" Margin="12,12,0,0" Name="label1" VerticalAlignment="Top" Width="376" FontWeight="Bold" FontSize="24" Content="Projects" /> <Slider Height="35" HorizontalAlignment="Left" Margin="158,553,0,0" Name="sliGridFontSize" VerticalAlignment="Top" Width="630" Value="11" Maximum="72" Minimum="11" /> <dataInput:Label Content="Font size" FontSize="20" FontWeight="Bold" Height="35" HorizontalAlignment="Left" Margin="12,553,0,0" Name="label2" VerticalAlignment="Top" Width="140" /> </Grid> </UserControl>
- Now, it is necessary to add code to retrieve data from the XML file and assign a value to the
ItemsSource
property of theDataGrid
control. First, you have to add a reference toSystem.Xml.Linq.dll
. Then, you can add the newInitializeGrid
method and call it from the class constructor as shown in the following lines forMainPage.xaml.cs.
using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Windows; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Shapes; // Added using System.Xml.Linq; namespace SilverlightProjects { Silverlight LOB RIAcreatingpublic partial class MainPage : UserControl { private void InitializeGrid() { XDocument docProjects = XDocument.Load("Projects.xml"); var projectsData = from el in docProjects.Descendants("project") select new Project { ProjectId = Convert.ToInt32(el.Attribute("projectId").Value), Title = Convert.ToString(el.Element("title").Value), EstimatedDaysLeft = Convert.ToInt32(el.Element("estimatedDaysLeft").Value), Status = Convert.ToString(el.Element("status").Value), AssignedTo = Convert.ToString(el.Element("assignedTo").Value), NumberOfTasks = Convert.ToInt32(el.Element("numberOfTasks").Value) }; dataGridProjects.ItemsSource = projectsData; } public MainPage() { InitializeComponent(); InitializeGrid(); } } }
- Build and run the solution. The default web browser will appear showing a grid with headers and the five rows defined in the previously added XML file, as shown in the following screenshot:
It is a very simple Silverlight LOB RIA displaying a data grid with rows that are read from the XML file included in the project, Projects.xml
.
First, we added the XML file with the definitions for the five projects. Then, we added a class with the necessary properties to hold the values defined in this XML file.
The InitializeGrid
method loads the projects from the Projects.xml
XML file (embedded and compressed in the .xap
file).
XDocument docProjects = XDocument.Load("Projects.xml");
Then, it uses a LINQ to XML query to create instances of the Project
class and assign values to their properties. Finally, it assigns this query to the ItemsSource
property of the DataGrid:
dataGridProjects.ItemsSource = projectsData;
Note
C# 3.0 (Visual C# 2008) introduced LINQ and it is very useful for processing queries for many different data sources. The features of LINQ and its usage in real-life scenarios are described in depth in LINQ Quickly (A Practical Guide to Programming Language Integrated Query with C#) by N. Satheesh Kumar from Packt Publishing.