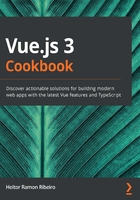
Using the reactivity and observable API outside the scope of Vue
In Vue 3, with the exposed APIs, we can use the Vue reactivity and reactive variables without the need to create a Vue application. This enables backend and frontend developers to take full advantage of the Vue reactivity API within their application.
In this recipe, we will create a simple JavaScript animation using the reactivity and watch APIs.
How to do it...
Here, we will create an application using the Vue exposed reactivity API to render an animation on the screen:
- Using the base example from the 'Creating the base file' section, create a new file named reactivity.html and open it.
- In the <head> tag, add a new <meta> tag with the attribute chartset defined as "utf-8":
<meta charset="utf-8"/>
- In the <body> tag, remove the div#app HTML element, and create a div HTML element with the id defined as marathon and the style attribute defined as "font-size: 50px;":
<div
id="marathon"
style="font-size: 50px;"
>
</div>
- In the empty <script> HTML element, create the constants of the functions that will be used using the object destructuring method, calling the reactivity and watch methods from the Vue global constant:
const {
reactive,
watch,
} = Vue;
- Create a constant named mod, defined as a function, which receives two arguments, a and b. This then returns an arithmetic operation, a modulus b:
const mod = (a, b) => (a % b);
- Create a constant named maxRoadLength with the value 50. Then, create a constant named competitor with the value as the reactivity function, passing a JavaScript object as the argument, with the position property defined as 0 and speed defined as 1:
const maxRoadLength = 50;
const competitor = reactive({
position: 0,
speed: 1,
});
- Create a watch function, passing an anonymous function as the argument. Inside the function, do the following:
- Create a constant named street, and define it as an Array with a size of maxRoadLength, and fill it with '_'.
- Create a constant named marathonEl, and define it as the HTML DOM node, #marathon.
- Select the element on the street in the array index of competitor.position and define it as "
" if the competitor.position number is even, or "
" if the number is odd.
- Define marathonEl.innertHTML as "" and street.reverse().join(''):

- Create a setInterval function, passing an anonymous function as the argument. Inside the function, define competitor.position as the mod function, passing competitor.position plus competitor.speed as the first argument, and maxRoadLength as the second argument:
setInterval(() => {
competitor.position = mod(competitor.position +competitor.speed,
maxRoadLength)
}, 100);
How it works...
Using the exposed reactive and watch APIs from Vue, we were able to create an application with the reactivity present in the Vue framework, but without the use of a Vue application.
First, we created a reactive object, competitor, that works in the same way as the Vue data property. Then, we created a watch function, which works in the same way as the watch property, but is used as an anonymous function. In the watch function, we made the road for the competitor to run on, and created a simple animation, using two different emojis, changing it based on the position on the road, so that it mimics an animation on the screen.
Finally, we printed the current runner on the screen and created a setInterval function of every 100ms to change the position of the competitor on the road: