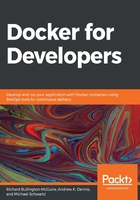
Preface
Software engineering teams are rapidly adopting containers to package and deploy their software. Providing a platform-agnostic experience, containers allow you to run applications with a variety of operating system images and to deploy on-premises, in data centers, and in the cloud. In order to support container-based applications, vendors have developed a wide variety of tools, ranging from Docker and Google's Kubernetes project to Lyft's Envoy service mesh and Netflix's Spinnaker. Whether you are working on the software development side of the house, hosting, and infrastructure, or constructing DevOps pipelines, you need both a broad and in-depth understanding of many concepts in order to manage container-based environments.
In Docker for Developers, we will start with a walk-through of the basics of developing with containers locally using Docker, and then move on to deploying production-ready, cloud-hosted systems with AWS. If you are interested in learning about container orchestration, deployment, monitoring, and security, then we think you will enjoy this book.
Who this book is for
Docker for Developers is geared toward engineers and DevOps personnel who want to learn the basics of containers and then build upon this knowledge to understand how to use containers in production, through a set of successively more sophisticated deployments. We will demonstrate how Docker applications can be deployed via CI/CD pipelines and managed in a production-grade, cloud-hosted environment. A basic understanding of containers would be helpful when tackling the book's subject matter, but this is not essential. It is assumed that readers of this book are familiar with Linux, the use of command-line tools, and basic software engineering concepts, such as version control and using Git.
What this book covers
Chapter 1, Introduction to Docker, provides some background on Docker, a walk-through of containers and their purpose, and presents the reader with an introduction to the topics that will be discussed in the book.
Chapter 2, Using VirtualBox and Docker Containers for Development, guides the reader through using a virtual machine locally for development and then compares this to how Docker can be used for containerized development projects.
Chapter 3, Sharing Containers Using Docker Hub, introduces the reader to Docker Hub and pre-built containers. Next, we explore the process of building specialized containers.
Chapter 4, Composing Systems Using Containers, investigates more complex situations where multiple containers need to work together as a complete system. Additionally, we give the reader an overview of Docker Compose.
Chapter 5, Alternatives for Deploying and Running Containers in Production, helps the reader understand the spectrum of choices when it comes to running containers in a production environment, including cloud options, on-premises and hybrid solutions.
Chapter 6, Deploying Applications with Docker Compose, discusses how to deploy a production application on a single host with Docker Compose and how to deal with logging and monitoring, along with the pros and cons of this simple setup.
Chapter 7, Continuous Deployment with Jenkins, shows how to use Jenkins for continuous integration (CI) and continuous deployment (CD) for containers, using a Jenkinsfile and multiple development branches.
Chapter 18, Deploying Docker Apps to Kubernetes, explores Kubernetes concepts, cloud distribution options, and shows how to create an Amazon Web Services Elastic Kubernetes Service (EKS) cluster for deploying Docker applications to Kubernetes.
Chapter 9, Cloud-Native Continuous Deployment Using Spinnaker, builds upon the skills we developed around CI/CD by integrating Netflix's Spinnaker with Kubernetes and looking at automated tests.
Chapter 10, Monitoring Docker Using Prometheus, Grafana, and Jaeger, explains how to monitor container-based applications using AWS CloudWatch, Prometheus, and Grafana. We introduce the OpenTracing API and implement it using Jaeger.
Chapter 11, Scaling and Load Testing Docker Applications, explores how to scale a Docker-based application through Kubernetes. It introduces the concept of a service mesh and shows a simple implementation using Envoy, integrating load balancing and advanced traffic routing and filtering, including utilization of the circuit breaker pattern. Finally, we show how to use k6.io to perform load testing to demonstrate that our application can scale out.
Chapter 12, Introduction to Container Security, walks the reader through basic container security concepts, including how virtualization and hypervisor security models work.
Chapter 13, Docker Security Fundamentals and Best Practices, builds upon the previous chapter's introduction and delves deeper into Docker and security components. This includes a comparison of Docker commands and their security implications.
Chapter 14, Advanced Docker Security – Secrets, Secret Commands, Tagging, and Labels, covers the topics of secrets, including passwords, and how they can be used securely with container-based environments. The reader is also introduced to the use of tagging and labeling best practices.
Chapter 15, Scanning, Monitoring, and Using Third-Party Tools, expands upon our logging and monitoring skills acquired from other chapters by refocusing on these elements from a security focus. Here, we also look at what options are available for users of AWS, Azure, and GCP and how we can scan containers for security issues using Anchore.
Chapter 16, Conclusion – End of the Road, but not the Journey, wraps the book up by revisiting what we have learned so far. Finally, we provide some ideas for where the reader can go next in exploring container-based projects. This ranges from adding Netflix Chaos Monkey to their CI/CD pipeline, to running Metasploit in a container.
To get the most out of this book
You will need a Windows, Mac, or Linux workstation that can run Docker. You should use the latest version if possible. Additionally, in order to complete any of the cloud-based projects, you will need to set up a cloud provider account. The examples use Amazon Web Services (AWS), although you could adapt much of the content to services hosted by another cloud provider:


While we do not explicitly demonstrate how to deploy the projects listed in this book to Microsoft Azure or the Google Cloud Platform, if you wish to explore some of the security features available on those cloud platforms, or try out the existing projects in them, you will need to create an account for each provider.
If you are using the digital version of this book, we advise you to type the code yourself or access the code via the GitHub repository (link available in the next section). Doing so will help you avoid any potential errors related to the copying and pasting of code.
Download the example code files
You can download the example code files for this book from your account at www.packt.com. If you purchased this book elsewhere, you can visit www.packtpub.com/support and register to have the files emailed directly to you.
You can download the code files by following these steps:
- Log in or register at www.packt.com.
- Select the Support tab.
- Click on Code Downloads.
- Enter the name of the book in the Search box and follow the onscreen instructions.
Once the file is downloaded, please make sure that you unzip or extract the folder using the latest version of:
- WinRAR/7-Zip for Windows
- Zipeg/iZip/UnRarX for Mac
- 7-Zip/PeaZip for Linux
The code bundle for the book is also hosted on GitHub at https://github.com/PacktPublishing/Docker-for-Developers. In case there's an update to the code, it will be updated on the existing GitHub repository.
We also have other code bundles from our rich catalog of books and videos available at https://github.com/PacktPublishing/. Check them out!
Code in Action
Code in Action videos for this book can be viewed at https://bit.ly/3kDmrtq.
Download the color images
We also provide a PDF file that has color images of the screenshots/diagrams used in this book. You can download it here: http://www.packtpub.com/sites/default/files/downloads/9781789536058_ColorImages.pdf.
Conventions used
There are a number of text conventions used throughout this book.
Code in text: Indicates code words in text, container names, folder names, filenames, file extensions, pathnames, dummy URLs, and user input. Here is an example: "This file needs to be added to the conf.d directory on the host."
A block of code or Dockerfile is set as follows:
FROM ubuntu:bionic
RUN apt-get -qq update && \
apt-get -qq install -y nodejs npm > /dev/null
RUN mkdir -p /app/public /app/server
COPY src/package.json* /app
WORKDIR /app
RUN npm -s install
When we wish to draw your attention to a particular part of a code block, the relevant lines or items are set in bold:
FROM alpine:20191114
RUN apk update && \
apk add nodejs nodejs-npm
RUN addgroup -S app && adduser -S -G app app
RUN mkdir -p /app/public /app/server
ADD src/package.json* /app/
Any command-line input or output is written as follows:
$ cp docker_daemon.yaml /path/to/conf.d/
$ vim /path/to/conf.d/conf.yaml
Bold: Indicates a new term, an important word, or words that you see on screen. For example, words in menus or dialog boxes appear in the text like this. Here is an example: "You can do this by clicking the Get It Now button on the Azure Marketplace website."
Tips or important notes
Appear like this.
Get in touch
Feedback from our readers is always welcome.
General feedback: If you have questions about any aspect of this book, mention the book title in the subject of your message and email us at customercare@packtpub.com.
Errata: Although we have taken every care to ensure the accuracy of our content, mistakes do happen. If you have found a mistake in this book, we would be grateful if you would report this to us. Please visit www.packtpub.com/support/errata, selecting your book, clicking on the Errata Submission Form link, and entering the details.
Piracy: If you come across any illegal copies of our works in any form on the internet, we would be grateful if you would provide us with the location address or website name. Please contact us at copyright@packt.com with a link to the material.
If you are interested in becoming an author: If there is a topic that you have expertise in, and you are interested in either writing or contributing to a book, please visit authors.packtpub.com.
Reviews
Please leave a review. Once you have read and used this book, why not leave a review on the site that you purchased it from? Potential readers can then see and use your unbiased opinion to make purchase decisions, we at Packt can understand what you think about our products, and our authors can see your feedback on their book. Thank you!
For more information about Packt, please visit packt.com.