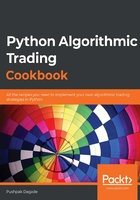
Expiry of financial instruments
Financial instruments may or may not have a fixed expiry date. If they do, they are last available for trading on their expiry date. Typically, instruments from a cash segment do not expire, whereas derivative instruments (those from the futures and options segment) have a short validity period, and expire on the given date. This recipe shows both types of instruments and how their expiry date can be fetched. An expiry date is static data, meaning it doesn't change during the live market hours.
Getting ready
Make sure the broker_connection and instruments objects are available in your Python namespace. Refer to the Technical requirements section of this chapter to set up broker_connection. Refer to the first recipe of this chapter to set up instruments.
How to do it…
We execute the following steps for this recipe:
- Get an instrument object using broker_connection:
>>> instrument1 = broker_connection.get_instrument('NSE',
'TATASTEEL')
- Check and print whether instrument1 will expire:
>>> print(f'Instrument expires: {instrument1.will_expire()}')
We get the following output:
Instrument expires: False
- Get another instrument object using broker_connection:
>>> instrument2 = broker_connection.get_instrument('NFO-FUT',
TATASTEEL20AUGFUT)
You shouldn't get any output here. This implies you have successfully fetched the instrument.
Please note that if you get the following output for this step, even after typing it correctly, please try this step with the latest available NFO-FUT segment script by referring to the table from the output in the Fetching the list of financial instruments recipe of this chapter:
ERROR: Instrument not found. Either it is expired and hence not available, or you have misspelled the "segment" and "tradingsymbol" parameters.
This can happen because the instrument, with tradingsymbol TATASTEEL20AUGFUT, was available at the time of writing this book, but has since expired and so isn't available anymore.
- Check and print whether instrument2 will expire:
>>> print(f'Instrument expires: {instrument2.will_expire()}')
We get the following output:
Instrument expires: True
- Print the expiry date of instrument2:
>>> print(f'Expiry date: {instrument2.expiry}')
We get the following output (your output may differ):
Expiry date: 2020-08-27
How it works…
Step 1 uses the get_instrument() method of the BrokerConnectionZerodha class to fetch an instrument and assign it to a new attribute, instrument1. This object is an instance of the Instrument class. The two parameters needed to call get_instrument are the exchange (NSE) and the trading symbol (TATASTEEL). In step 2, we check whether the instrument will expire using the will_expire() method. The output of this step is False. We repeat the same procedure in steps 3 and 4, this time for a different instrument, assigned to a new attribute, instrument2, which gives an output of True for the will_expire() method. This is shown in the output of step 4. Finally, in step 5, we fetch the expiry date of instrument2 using the expiry attribute.