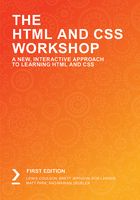
CSS
Cascading Style Sheets (CSS) is a style sheet language used to describe the presentation of a web page. The language is designed to separate concerns. It allows the design, layout, and presentation of a web page to be defined separately from content semantics and structure. This separation helps keeps source code readable and it is important because a designer can update styles separately from a developer who is creating the page structure or a web editor who is changing content on a page.
A set of CSS rules in a style sheet determines how an HTML document is displayed to the user. It can determine whether elements in the document are rendered at all, whether they appear in some context but not others, how they are laid out on the web page, whether they are rendered in a different order to the order in which they appear within a document, and their aesthetic appearance.
We will begin by looking at the syntax of CSS.
Syntax
A CSS declaration is made of two parts: a property and a value. The property is the name for some aspect of style you want to change; the value is what you want to set it to.
Here is an example of a CSS declaration:
color: red;
The property is color and the value is red. In CSS, color is the property name for the foreground color value of an element. That essentially means the color of the text and any text decoration (such as underline or strikethrough). It also sets a currentcolor value.
For this declaration to have any effect on an HTML document, it must be applied to one or more elements in the document. We do this with a selector. For example, you can select all the <p> elements in a web page with the p selector. So, if you wanted to make the color of all text in all paragraph elements red, you would use the following CSS ruleset:
p {
color: red;
}
The result of this CSS ruleset applied to an HTML document can be seen in the following figure:

Figure 1.18: Result of a CSS rule applied to <p> elements in HTML
The curly braces represent a declaration block and that means more than one CSS declaration can be added to this block. If you wanted to make the text in all paragraph elements red, bold, and underlined, you could do that with the following ruleset:
p {
color: red;
font-weight: bold;
text-decoration: underline;
}
The result of this CSS ruleset applied to an HTML document can be seen in the following figure:

Figure 1.19: Several CSS declarations applied to <p> elements in HTML
Multiple selectors can share a CSS ruleset. We can target these with a comma-separated list. For example, to apply the color red to p elements, h1 elements, and h2 elements, we could use the following ruleset:
p, h1, h2 {
color: red;
}
Multiple CSS rulesets form a style sheet. The order of these CSS rules in a style sheet is very important as this is partly how the cascade or specificity of a rule is determined. A more specific rule will be ranked higher than a less specific rule and a higher-ranked rule will be the style shown to the end user. We will look at cascade and specificity later in this chapter:

Figure 1.20: A CSS ruleset explained
Adding Styles to a Web Page
There are several ways to add your styles to a web page:
- You can use inline styles, which are applied directly to an element in the HTML document using the style attribute. The value of the style attribute is a CSS declaration block, meaning you can apply a semicolon-separated list of CSS declarations to the element.
- You can use a style element to add style information to an HTML document. The style element can be a child of either the head element or body element of a document. The head element tends to be preferable as the styles will be applied to your page more quickly.
- You can provide a style sheet as an external resource using the link element. One of the rationalities behind style sheets is the separation of concerns, which is why this approach is often recommended.
We will try out each of these methods in the following exercises.
Exercise 1.05: Adding Styles
In this exercise, we will be styling web pages by adding styles within the HTML document itself.
Here are the steps:
- Open the chapter_1 folder in VSCode (File > Open Folder…) and we will create a new plain text file by clicking File > New File. Then, save it in HTML format, by clicking File > Save As... and enter the File name: Adding styles.html and start with the following web page:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Adding styles</title>
</head>
<body>
<h1>Adding styles</h1>
<p>First paragraph</p>
<p>Second paragraph</p>
</body>
</html>
Without any styles, this web page would look like the following:
Figure 1.21: The unstyled web page
- We've decided to use a nicer font and make some of the text red. To do this, we will add a style element to the head element. We can add the following code under the title element:
<style>
h1 {
font-family: Arial, Helvetica, sans-serif;
font-size: 24px;
margin: 0;
padding-bottom: 6px;
}
p {
color: red;
}
</style>
The results of this code change are that we should now have styles applied to the h1 element and to both of the p elements, all of which will have red text. The result will look similar to the following figure:
Figure 1.22: The web page with styles applied
- Finally, we will give the first paragraph element a different style by overriding the style set in the head element. Let's add an inline style to the first paragraph, setting the color to blue and adding a line-through text decoration as follows:
<p style="color: blue; text-decoration: line-through">First paragraph</p>
If you now right-click on the filename in VSCode on the left-hand side of the screen and select open in default browser, you will see the following web page in your browser:
Figure 1.23: The web page with inline style applied
Something to note is that the inline style, applied to the first paragraph, takes precedence over the more general CSS rule applied to all p elements in the style element in the head element of the document. We will look at specificity and the rules of the cascade later in this chapter, but first, let's try moving these style rules into an external file.
Exercise 1.06: Styles in an External File
In this exercise, we will separate the concerns of presentation and structure of Exercise 1.05, Adding Styles, by moving all the styles to an external file.
The steps are as follows:
- Open the chapter_1 folder in VSCode (File > Open Folder…) and create a new plain text file by clicking File > New File. Then, save it in HTML format, by clicking File > Save As... and enter the File name: index-with-external-styles.html.Add the same web page as in Exercise 1.05, Adding Styles, to the file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Adding styles</title>
</head>
<body>
<h1>Adding styles</h1>
<p>First paragraph</p>
<p>Second paragraph</p>
</body>
</html>
- We will add a link element to reference a .css file below the title element:
<link href="styles/Exercise_1.06.css" rel="stylesheet">
- Next, we need to save a file named styles.css in the same directory as index-with-external-styles.html.
- Create a styles directory and add the following styles to Exercise_1.06.css:
h1 {
font-family: Arial, Helvetica, sans-serif;
font-size: 24px;
margin: 0;
padding-bottom: 6px;
}
p {
color: red;
}
- To get the equivalent styles that we had at the end of Exercise 1.05, Adding Styles, without using an inline style, we have to have a specific way of targeting the first p element. We will use the :first-of-type pseudo-class. You will learn more about CSS selectors later in this chapter. For now, add this CSS rule to the bottom of styles.css:
p:first-of-type {
color: blue;
text-decoration: line-through;
}
The result will be as seen in Figure 1.23 – the same result as in Exercise 1.05, Adding Styles. The difference is that we have removed all references to styles from the HTML document into their own external resources. We have successfully separated concerns.
Both these methods add styles to the HTML document when it loads. Similar to the HTML DOM, we can manipulate CSS programmatically with JavaScript. This is because the styles are also represented as an object model called the CSSOM.