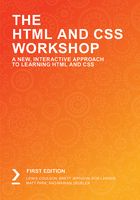
The Box Model
So far, all the elements on our pages look almost identical because we have not learned how to adjust the size of each element. We are now ready to progress to more realistic page designs by introducing a foundational layout concept called the box model.
Try to picture each HTML element as a box made up of different layers. The different layers are the element's content box, padding, border, and margin. We will explore each of these layers one by one. The following figure illustrates how all aspects of the box model relate to one another. You can see that the margin is the outermost part, followed by the element's border and padding between the border and content area:

Figure 2.19: The box model
We will now look at each of the box model elements, in turn, starting with the innermost content box.
Content Box
The content box is the part of the element where the actual content lives. This is typically text but could contain other child elements or media elements such as images. The most important CSS properties for this layer are width and height. As a developer, you would typically give these values expressed in pixels or percentages. The following code shows some example values, followed by the corresponding output figure for these properties:
width: 200px;
height: 100px;
In the following figure, we will see what the content area looks like after CSS is applied to the preceding code:

Figure 2.20: The content box
Next, we will work our way out to the next layer of the box model – padding.
The padding Property
The padding area is the layer that provides spacing between the content box and the border. The amount of spacing in this layer can be specified in all directions – top, right, bottom, and left. CSS provides a padding property where you can specify values for the amount of spacing in all directions. If you want to apply the same amount of padding in all directions, you can just give a single value. If you want to apply the same values for vertical and horizontal directions, you can specify two values. It also provides direction-specific properties – padding-top, padding-right, padding-bottom, and padding-left. The following code shows a number of example values for these properties:
/* 50px of padding applied in all directions */
padding: 50px;
/* 50px of padding applied vertically and 0px applied horizontally */
padding: 50px 0;
/* 10px of padding applied to the top */
padding-top: 10px;
/* 10px of padding applied to the right */
padding-right: 10px;
/* 10px of padding applied to the bottom */
padding-bottom: 10px;
/* 10px of padding applied to the left */
padding-left: 10px;
The following figure illustrates what the content and padding areas would look like after CSS is applied to the following code:
width: 200px;
height: 100px;
padding: 25px;

Figure 2.21: Padding
Now that we understand how the content and padding layers relate to one another, we will work our way out to the next layer of the box model – the border.
The border Property
The border area is the layer that sits between the end of the padding area and the beginning of the margin. By default, the border isn't visible; it can only be seen when you explicitly set a value that will allow you to see the border. Similar to the padding property, CSS provides a shorthand property called border, and also the direction-specific properties – border-top, border-right, border-bottom, and border-left. All of these properties require three values to be provided; the width of the border, the border style, and finally, the color of the border. The following code shows some example values for these properties:
/* border styles applied in all directions */
border: 5px solid red;
/* border styles applied to the top */
border-top: 5px solid red;
/* border styles applied to the right */
border-right: 15px dotted green;
/* border styles applied to the bottom */
border-bottom: 10px dashed blue;
/* border styles applied to the left */
border-left: 10px double pink;
The following figure illustrates how the four different border styles would appear if applied to an element:

Figure 2.22: Border styles
The content, padding, and border layers is obtained with the following code:
width: 200px;
height: 100px;
padding: 25px;
border: 10px solid black;
The following figure is the output for the preceding code:

Figure 2.23: Border
Now that we understand how the content, padding and margin layers relate to one another, we will work our way out to the final layer of the box model – the margin.
The margin Property
The margin area is the layer that provides spacing between the edge of the border and out toward other elements on the page. The amount of spacing in this layer can be specified in all directions – top, right, bottom, and left. The CSS provides a margin property where you can specify values for the amount of spacing in all directions. It also provides direction-specific properties – margin-top, margin-right, margin-bottom, and margin-left. The following code shows a number of example values for these properties:
margin: 50px;
margin: 50px 0;
margin-top: 10px;
margin-right: 10px;
margin-bottom: 10px;
margin-left: 10px;
The content, padding, border, and margin layers is obtained with the following code:
width: 200px;
height: 100px;
padding: 25px;
border: 10px solid black;
margin: 25px;
The following figure is the output for the preceding code:

Figure 2.24: Margin
To get some practice looking at how different HTML elements make use of the box model, you can use the webtools inspector in your favorite browser. In Chrome, you can inspect an element and investigate how the box model is used for each element. If you inspect an element and then click the Computed tab on the right-hand side, you will see a detailed view. The following figure shows an example of an element from the Packt website revealing the values for properties from the box model:

Figure 2.25: Chrome web tools box model inspection view
In the following exercise, we will play around with the different box model properties to get some practice with box model-related CSS properties.
Exercise 2.03: Experimenting with the Box Model
The aim of this exercise will be to create the three boxes as shown in the following output screenshot:

Figure 2.26: Expected boxes
The steps to complete the exercise are as follows:
- First, let's add the following HTML skeleton to a file called boxes.html in VSCode:
<!DOCTYPE html>
<html>
<head>
<title>Experimenting with the box model</title>
<style type="text/css">
</style>
</head>
<body>
<div class="box-1">Box 1</div>
<div class="box-2">Box 2</div>
<div class="box-3">Box 3</div>
</body>
</html>
- Now, let's add some CSS to the first box, observing the width, height, padding, and border properties we are adding. We will add the CSS in between the opening and closing style tags, as shown in the following code, to render the following figure:
<style type="text/css">
.box-1 {
float: left;
width: 200px;
height: 200px;
padding: 50px;
border: 1px solid red;
}
</style>
The following figure shows the output of the preceding code:
Figure 2.27: Output for box 1
- Now, let's add the CSS to the second box in Figure 2.25, observing how the width, height, padding, and border properties differ from the first box. We are using percentage-based measurements for the width and height properties, as shown in the following code:
.box-2 {
float: left;
width: 20%;
height: 20%;
padding-top: 50px;
margin-left: 10px;
border: 5px solid green;
}
The following figure shows the output of the preceding code:
Figure 2.28: Output for boxes 1 and 2
- Finally, let's add the CSS to the third box in Figure 2.25, observing how the width, height, padding, and border properties differ from the first and second boxes, as shown in the following code, to render the following figure:
.box-3 {
float: left;
width: 300px;
padding: 30px;
margin: 50px;
border-top: 50px solid blue;
}
The following figure shows the output of the preceding code:

Figure 2.29: Output for boxes 1, 2, and 3
If you now right-click on the filename in VSCode on the left-hand side of the screen and select open in default browser, you will see the web page in your browser.
This should give you a sense of what's possible with the box model. Feel free to change the various different properties and experiment with different combinations.
Putting It All Together
We now know how to correctly markup a web page with the correct HTML5 structural tags. We also know how to use the three most popular CSS layout techniques. Finally, we have an understanding of how the box model works. We will now build the two complete web pages, combining all of the things we have learned so far in this chapter.
Exercise 2.04: Home Page Revisited
In this exercise, we will be using the wireframe in Figure 2.13 for a home page design used in Activity 2.01, Video Store Home Page. We will build a version of this page, incorporating the concepts from the box model topic. Our aim will be to build a page as shown in the wireframe Figure 2.15:
The steps to complete this exercise are as follows:
- Create a new file called home.html in VSCode.
- Use the following HTML code as a start file. Again, don't worry if some of the CSS doesn't make sense to you. We will look into this part of the styling in more detail in Chapter 3, Text and Typography:
<!DOCTYPE html>
<html>
<head>
<title>Video store home page</title>
<style>
header,
nav,
section,
footer {
background: #659494;
border-radius: 5px;
color: white;
font-family: arial, san-serif;
font-size: 30px;
text-align: center;
}
header:before,
nav:before,
section:before,
footer:before {
content: '<';
}
header:after,
nav:after,
section:after,
footer:after {
content: '>';
}
</style>
</head>
<body>
<!-- your code goes here -->
</body>
</html>
- Now, let's add some styling for the structural elements. Notice how we have used what we have learned from The Box Model topic to include border, padding, and margin with our structural elements. We will use a border to visually define the outer edge of the element, along with some padding to add spacing between the text and the outer edge of the element and a bottom margin to provide vertical spacing between the elements. We will add this just before the closing style tag:
/* CSS code above */
header,
nav,
section,
footer {
border: 1px solid gray;
padding: 50px;
margin-bottom: 25px;
}
</style>
If you now right-click on the filename in VSCode on the left-hand side of the screen and select open in default browser, you will see the web page in your browser as shown in the following figure:

Figure 2.30: Output of home page
You should see a web page resembling the one shown in the home page wireframe.
Exercise 2.05: Video Store Product Page Revisited
In this exercise, we will be using the wireframe for a product page design as in Figure 2.15. We will build a more realistic version incorporating the box model. Our aim will be to build a page as shown in the wireframe Figure 2.15.
The steps to complete the exercise are as follows:
- Create a new file called product.html in VSCode with the following code:
<!DOCTYPE html>
<html>
<head>
<title>Video store product page</title>
<style>
</style>
</head>
<body>
</body>
</html>
- In order to add styling, add the following code in between the style tags:
header, nav, section, footer {
background: #659494;
border-radius: 5px;
color: white;
font-family: arial, san-serif;
font-size: 30px;
text-align: center;
}
header:before, nav:before, footer:before {
content: '<';
}
header:after, nav:after, footer:after {
content: '>';
}
- We will now add the HTML for the page elements, which are header, nav, section, and footer. The product items will be div elements inside the section element, as shown in the following code:
<body>
<header>header</header>
<nav>nav</nav>
<section>
<div>product 1</div>
<div>product 2</div>
<div>product 3</div>
<div>product 4</div>
<div>product 5</div>
<div>product 6</div>
<div>product 7</div>
<div>product 8</div>
</section>
<footer>footer</footer>
</body>
- Now, let's add some styling for the structural elements. This is the same code as in the previous exercise. We will use a border to visually define the outer edge of the element, along with some padding to add spacing between the text and the outer edge of the element and a bottom margin to provide vertical spacing between elements. Again, we will add the CSS just before the closing style tag:
/* CSS code above */
header,
nav,
section,
footer {
border: 1px solid gray;
padding: 20px;
margin-bottom: 25px;
}
</style>
- We will now need to add some styling for the product cards. We will use the grid layout technique, as this will allow our code to be as concise as possible:
/* CSS code above */
section {
display: grid;
grid-template-columns: auto auto auto auto;
}
section div {
border: 2px solid white;
padding: 30px;
margin: 10px;
}
</style>
If you now right-click on the filename in VSCode on the left-hand side of the screen and select open in default browser, you will see the web page in your browser as shown in the following figure:

Figure 2.31: Output for the video store product page
You should now see a web page resembling the one shown in the product page wireframe.
Activity 2.02: Online Clothes Store Home Page
Suppose you are a freelance web designer/developer and have just landed a new client. For your first project, the client wants a web home page developed for their online clothes store.
Using the skills learned in this chapter, design and develop the home page layout for the new online store.
The steps are as follows:
- Produce a wireframe, either by hand or by using a graphics tool, for the new home page layout.
- Create a file named home.html in VSCode.
- Start writing the markup for the page.
- Now, style the layout with CSS.
The following figure shows the expected output for this activity:

Figure 2.32: Expected output for the online clothes store home page
Note
The solution to this activity can be found on page 582.