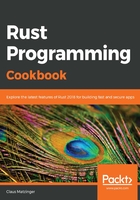
How it works...
Enums in Rust encapsulate choices—just as in any language. However, they behave similarly to regular structures in a lot of ways:
- They can have impl blocks for traits and functions.
- Unnamed and named properties can carry different values.
These aspects make them really great candidates for choices of all kinds, be it configuration values, flags, constants, or wrapping errors, as we did in Step 2. Typical enums in other languages map a name to a numerical value of your choice, but Rust goes one step further. Instead of just numerical values, Rust's enum can have any value and even named properties. Take a look at the definition in Step 2:
pub enum ApplicationError {
Code { full: usize, short: u16 },
Message(String),
IOWrapper(io::Error),
Unknown
}
ApplicationError::Code features two properties, one called full and one called short—assignable just like any other struct instance. The second and third variations, Message and IOWrapper, encapsulate another type instance entirely, one a String, and the other an std::io::Error, similar to tuples.
The additional ability to work in match clauses makes these constructs very useful, especially for large code bases where readability is important—an example of which can be found in Step 3, where we implement a function at the enum's type. This function maps explicit enum instances to strings for easier printing.
Step 4 implements a helper function that provides us with different kinds of errors and values to work with, something that we require in Step 5, where we create two extensive tests of these functions. There, we use the match clause (which will also be discussed in a later recipe in this chapter) to extract values from the errors and match on multiple enum variants in a single arm. Additionally, we created a test to show that the print_kind() function works by using a Vec as a stream (thanks to it implementing the Write trait).
We've successfully learned how to create meaningful numbers with enums. Now, let's move on to the next recipe.