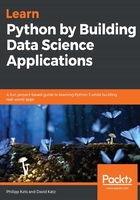
Enumerations
Enumerations are somewhat less popular data structures in Python. They are quite similar to named tuples, as they are immutable and their attributes are named. Enumerations also have one interesting property: they are always singletons. In other words, you can't have more than one instance of an Enum class at the same time. With that, enumerations work well as semantic layers (aliases) for a set of specific values; for example, for categories, we can define colors in the palette, aliasing specific implementation. The singleton property means that the value will always be exactly the same throughout the code.
In the following example, we define an Enum class for the color theme. Our Enum object supports three colors: MAIN, SECONDARY, and ACCENT. Note how we alias the values:
from enum import Enum
>>> class Colors(Enum): ... MAIN = 'darkblue' ... SECONDARY = 'lightgrey' ... ACCENT = 'teal'
As a result, we have a clear representation of the color palette (theme) in the code. Now, we can access the values without explicitly stating them. In the following code, we define a function that generates a div HTML object that is colored with the SECONDARY color. This level of semantics allows us to swap color themes later, with no need to adjust the code. Note that we don't need to create an instance of the class here:
def mainblock(content):
return f"<div style='color:{Colors.SECONDARY.value}'>{content}</div>"
>>> mainblock('Hello!')
<div style='color:lightgrey'>Hello!</div>
While Enum can be useful in certain cases, it was rarely used in code until recently. With the new feature of a type annotation being introduced in Python 3, enumerations have turned out to be a useful structure to represent an argument to be within a set of possible values. In particular, we will use Enum as a type hint in Chapter 18, Serving Models with a RESTful API, where it will help us to define a correct endpoint for the API we will build.
Next, after data structures, let's move on to generators and see what they are like.