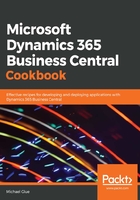
上QQ阅读APP看书,第一时间看更新
How to do it...
- Open your AL project folder in Visual Studio Code.
- First, we need to create the new logic that we will implement.
In Visual Studio Code's Explorer pane, right-click and create a new file named Check Customer Television Shows.al. In the Editor tab, add the following code, which checks to make sure that there is at least one television show defined as the favorite for the given customer:
codeunit 50100 "Check Cust. Television Shows"
{
procedure CheckCustomerTelevisionShows(CustomerNo:
Code[20])
begin
ValidateFavoriteShowExists(CustomerNo);
end;
local procedure ValidateFavoriteShowExists(CustomerNo:
Code[20])
var
CustomerTelevisionShow: Record "Customer Television
Show";
NoFavoriteShowErr: Label 'You need to define a favorite
television show for Customer %1.';
begin
CustomerTelevisionShow.SetRange("Customer No.",
CustomerNo);
CustomerTelevisionShow.SetRange(Favorite, true);
if CustomerTelevisionShow.IsEmpty() then
Error(NoFavoriteShowErr, CustomerNo);
end;
}
Use the tcodeunit snippet to create a new codeunit object, and the tprocedure snippet to create new functions.
- Now that we have our new logic, let's hook it into the sales posting routine. In Visual Studio Code's Explorer pane, right-click and create a new file named Sales Post Subscribers.al. In the Editor tab, add the following code:
codeunit 50101 SalesPostSubscribers
{
[EventSubscriber(ObjectType::Codeunit, Codeunit::"Sales-Post
(Yes/No)", 'OnAfterConfirmPost', '', false, false)]
local procedure OnAfterConfirmPost(SalesHeader: Record "Sales
Header")
var
CheckCustomerTelevisionShows: Codeunit "Check Cust.
Television Shows";
begin
CheckCustomerTelevisionShows.CheckCustomerTelevisionShows
(SalesHeader."Sell-to Customer No.");
end;
}
Use the teventsub snippet to create new event subscribers.
- Time to test! Press F5 to build and publish your application. When your browser opens and you log in to your sandbox, perform the following steps:
- Use the
icon and search for sales invoices to go to the Sales Invoices page.
- Select an existing sales invoice from the list or create a new one:
- Make sure you use an invoice for a customer that has no television shows defined!
- Post the sales invoice.
- Use the
After confirming that you want to post the document, you should see an error similar to the following one:

If you want to test further, repeat the preceding process but before you post the invoice, add at least one television show to the customer and mark it as a favorite. Your sales invoice should then post without any error.
For superior performance, try to keep your subscriber codeunits as small as possible. This minimizes the time it takes for the system to load those codeunits into memory when the events are fired. You may also want to consider making your subscribers single instance so they only need to be loaded once per session.