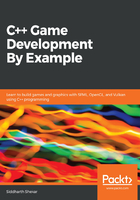
Operators
An operator is a symbol that performs a certain operation on a variable or expression. So far, we have used the = sign, which calls an assignment operator that assigns a value or expression from the right-hand side of the equals sign to a variable on the left-hand side.
The simplest form of other kinds of operators are arithmetic operators such as +, -, *, /, and %. These operators operate on a variable such as int and float. Let's look at some of the use cases of these operators:
#include <iostream> #include <conio.h> // Program prints out value of a + b and x + y to screen int main() { int a = 8; int b = 12; std::cout << "Value of a + b is : " << a + b << std::endl; float x = 7.345f; float y = 12.8354; std::cout << "Value of x + y is : " << x + y << std::endl; _getch(); return 0; }
The output of this is as follows:

Let's look at examples for other operations as well:
#include <iostream> #include <conio.h> // Program prints out values to screen int main() { int a = 36; int b = 5; std::cout << "Value of a + b is : " << a + b << std::endl; std::cout << "Value of a - b is : " << a - b << std::endl; std::cout << "Value of a * b is : " << a * b << std::endl; std::cout << "Value of a / b is : " << a / b << std::endl; std::cout << "Value of a % b is : " << a % b << std::endl; _getch(); return 0; }
The output is as shown as follows:

+, -, *, and / are self-explanatory. However, there is one more arithmetic operator: %, which is called the module operator. It returns the remainder of a division .
How many times is 5 contained in 36? 7 times with a remainder of 1. That's why the result is 1.
Apart from the arithmetic operator, we also have an increment/decrement operator.
In programming, we increment variables often. You can do a=a+1; to increment and a=a-1; to decrement a variable value. Alternatively, you can even do a+=1; and a-=1; to increment and decrement, but in C++ programming there is an even shorter way of doing that, which is by using the ++ and -- signs to increment and decrement the value of a variable by 1.
Let's look at an example of how to use it to increment and decrement a value by 1:
#include <iostream> #include <conio.h> // Program prints out values to screen int main() { int a = 36; int b = 5; std::cout << "Value of ++a is : " << ++a << std::endl; std::cout << "Value of --b is : " << --b << std::endl; std::cout << "Value of a is : " << a << std::endl; std::cout << "Value of b is : " << b << std::endl; _getch(); return 0; }
The output of this is as follows:

Consequently, the ++ or -- operator increments the value permanently. If the ++ is to the left of the variable, it is called a pre-increment operator. If it is put afterward, it is called a post-increment operator. There is a slight difference between the two. If we put the ++ on the other side, we get the following output:

In this case, a and b are incremented and decremented in the next line. So, when you print the values, it prints out the correct result.
It doesn't make a difference here, as it is a simple example, but overall it does make a difference and it is good to understand this difference. In this book, we will mostly be using post-increment operators.
In fact, this is how C++ got its name; it is an increment of C.
Apart from arithmetic, increment, and decrement operators, you also have logical and comparison operators, as shown:
Logical operators:

Comparison operators:

We will cover these operators in the next section.