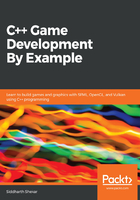
Iteration
Iteration is the process of calling the same statement repeatedly. C++ has three iteration statements: the while, do...while, and for statements. Iteration is also commonly referred to as loops.
The while loop syntax looks like the following:
while (condition) statement;
Let's look at it in action:
#include <iostream> #include <conio.h> // Program prints out values to screen int main() { int a = 10; int n = 0; while (n < a) { std::cout << "value of n is: " << n << std::endl; n++; } _getch(); return 0; }
Here is the output of this code:

Here, the value of n is printed to the console until the condition is met.
The do while statement is almost the same as a while statement except, in this case, the statement is executed first and then the condition is tested. The syntax is as follows:
do statement
while (condition);
You can give it a go yourself and check the result.
The loop that is most commonly used in programming is the for loop. The syntax for this looks as follows:
for (initialization; continuing condition; update) statement;
The for loop is very self-contained. In while loops, we have to initialize n outside the while loop, but in the for loop, the initialization is done in the declaration of the for loop itself.
Here is the same example as the while loop but with the for loop:
#include <iostream> #include <conio.h> // Program prints out values to screen int main() { for (int n = 0; n < 10; n++) std::cout << "value of n is: " << n << std::endl; _getch(); return 0; }
The output is the same as the while loop but look how compact the code is compared to the while loop. Also the n is scoped locally to the for loop body.
We can also increment n by 2 instead of 1, as shown:
#include <iostream> #include <conio.h> // Program prints out values to screen int main() { for (int n = 0; n < 10; n+=2) std::cout << "value of n is: " << n << std::endl; _getch(); return 0; }
Here is the output of this code:
