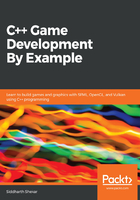
上QQ阅读APP看书,第一时间看更新
Switch statement
The last of the statements is the switch statement. A switch statement checks for several cases of values and if a value matches the expression, then it executes the corresponding statement and breaks out of the switch statement. If it doesn't find any of the values, then it will output a default statement.
The syntax for it looks as follows:
switch( expression){ case constant1: statement1; break; case constant2: statement2; break; . . . default: default statement; break; }
This looks very familiar to the else if statements, but this is more sophisticated. Here is an example:
#include <iostream> #include <conio.h> // Program prints out values to screen int main() { int a = 28; switch (a) { case 1: std::cout << " value of a is " << a << std::endl; break; case 2: std::cout << " value of a is " << a << std::endl; break; case 3: std::cout << " value of a is " << a << std::endl; break; case 4: std::cout << " value of a is " << a << std::endl; break; case 5: std::cout << " value of a is " << a << std::endl; break; default: std::cout << " value a is out of range " << std::endl; break; } _getch(); return 0; }
The output is as follows:

Change the value of a to equal 2 and you will see that it prints out the statement to when case 2 is correct.
Also note that it is important to add the break statement. If you forget to add it, then the program will not break out of the statement.