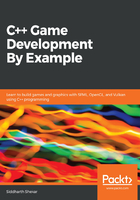
Enums
Enums are used for enumerating items in a list. When comparing items, it is easier to compare names rather than just numbers. For example, the days in a week are Monday to Sunday. In a program, we will assign Monday to 0, Tuesday to 1, and Sunday to 7, for example. To check whether today is Friday, you will have to count to and arrive at 5. However, wouldn't it be easier to just check if Today == Friday?
For this, we have enumerations, declared as follows:
enum name{ value1, value2, . . . };
So, in our example, it would be something like this:
#include <iostream> #include <conio.h> // Program prints out values to screen enum Weekdays { Monday = 0, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday, }; int main() { Weekdays today; today = Friday; if (today == Friday) { std::cout << "The weekend is here !!!!" << std::endl; } _getch(); return 0; }
The output of this is as follows:

Also note, here, Monday = 0. If we don't use initializers, the first item's value is set to 0. Each following item that does not use an initializer will use the value of the preceding item plus 1 for its value.