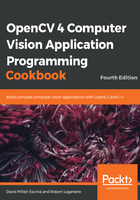
How it works...
Since Version 2.2, the OpenCV library has been divided into several modules. These modules are built-in library files located in the lib directory. Some of the commonly used modules are as follows:
- The opencv_core module that contains the core functionalities of the library, in particular, basic data structures and arithmetic functions
- The opencv_imgproc module that contains the main image-processing functions
- The opencv_highgui module that contains the image and video reading and writing functions along with some user interface functions
- The opencv_features2d module that contains the feature point detectors and descriptors and the feature point matching framework
- The opencv_calib3d module that contains the camera calibration, two-view geometry estimation, and stereo functions
- The opencv_video module that contains the motion estimation, feature tracking, and foreground extraction functions and classes
- The opencv_objdetect module that contains the object detection functions such as the face and people detectors
The library also includes other utility modules that contain machine learning functions (opencv_ml), computational geometry algorithms (opencv_flann), contributed code (opencv_contrib), and many more. You will also find other specialized libraries that implement higher level functions, such as opencv_photo for computational photography and opencv_stitching for image-stitching algorithms. There is also a new branch that contains other library modules, which include non-free algorithms, non-stable modules, or experimental modules. This branch is on the opencv-contrib GitHub branch. When you compile your application, you will have to link your program with the libraries that contain the OpenCV functions you are using, linking it with the opencv-contrib folder.
All these modules have a header file associated with them (located in the include directory). A typical OpenCV C++ code will, therefore, start by including the required modules. For example (and this is the suggested declaration style), it will look like the following code:
#include <opencv2/core/core.hpp> #include <opencv2/imgproc/imgproc.hpp> #include <opencv2/highgui/highgui.hpp>
You can download the example code files for all Packt books you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files emailed directly to you.
You might see an OpenCV code starting with the following command:
#include "cv.h"
This is because it used the old style before the library was restructured into modules and became compatible with older definitions.