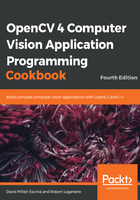
上QQ阅读APP看书,第一时间看更新
How to do it...
We are going to create a function for salt noise and how to use it. To do it, we are going to follow these steps:
- Create a function that receives an input image and a number. This is the image that will be modified by our function. The second parameter is the number of pixels on which we want to overwrite white values:
void salt(cv::Mat image, int n) {
- Define two variables that we are going to use to store a random position on an image:
int i,j;
- Create a loop that iterates n number of times. This is the second parameter and defines the number of pixels on which we want to overwrite white values:
for (int k=0; k<n; k++) {
- Generate two random image positions using the std::rand() function. We store the x value on an i variable and the y value on j. The std::rand() function returns a value between 0 and RAND_MAX, then we apply a module % to it with cols or rows of the image to return only values between 0 and the width or height:
// rand() is the random number generator i= std::rand()%image.cols; j= std::rand()%image.rows;
- Using the type method, we distinguish between the two cases of gray-level and color images. In the case of a gray-level image, the number 255 is assigned to the single 8-bit value using the Mat function at<type>(y,x):
if (image.type() == CV_8UC1) { // gray-level image image.at<uchar>(j,i)= 255; }
- For a color image, you need to assign 255 to the three primary color channels in order to obtain a white pixel. To access each channel, we can use the array access (nChannel) where nChannel is the number of channels, 0 is blue, 1 is green, and 2 is blue in the BGR format:
else if (image.type() == CV_8UC3) { // color image image.at<cv::Vec3b>(j,i)[0]= 255; image.at<cv::Vec3b>(j,i)[1]= 255; image.at<cv::Vec3b>(j,i)[2]= 255; }
- Finally, remember to close the loop and function brackets:
} }
- To use this function, we can read an image from our disk:
// open the image cv::Mat image= cv::imread("boldt.jpg");
- Now call the function using the image loaded and a number of pixels to change, for example, 3000:
// call function to add noise salt(image,3000);
- Finally, display the image using the cv::imshow function:
// display image cv::imshow("Image",image);
The resulting image will look as follows, as shown in this screenshot:

Let's see how the preceding instructions work when we execute them.