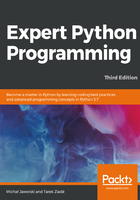
Syntax changes
Syntax changes that make it difficult for the existing code to run are the easiest to spot—they will cause the code to not run at all. The Python 3 code that uses new syntax elements will fail to run on Python 2 and vice versa. The elements that were removed from official syntax will make Python 2 code visibly incompatible with Python 3. Any attempt to run the code that has such issues will immediately cause the interpreter to fail, raising a SyntaxError exception. Here is an example of the broken script that has exactly two statements, of which none will be executed due to the syntax error:
print("hello world") print "goodbye python2"
Its actual result when run on Python 3 is as follows:
$ python3 script.py File "script.py", line 2 print "goodbye python2" ^ SyntaxError: Missing parentheses in call to 'print'
When it comes to new elements of Python 3 syntax, the total list of differences is a bit long, and any new Python 3.x release may add new elements of syntax that will raise such errors on earlier releases of Python (even on the same 3.x branch). The most important of them are covered in Chapter 2, Modern Python Development Environments, and Chapter 3, Modern Syntax Elements – Below the Class Level, so there is no need to list all of them here.
The list of things that used to work in Python 2 that will cause syntax or functional errors in Python 3 is shorter. Here are the most important backwards incompatible changes:
- print is no longer a statement, but a function, so the parenthesis is now obligatory.
- Catching exceptions changed from except exc, var to except exc as var.
- The <> comparison operator has been removed in favor of !=.
- from module import * (https://docs.python.org/3.0/reference/simple_stmts.html#import) is now allowed only on module level, and no longer inside the functions.
- from .[module] import name is now the only accepted syntax for relative imports. All imports not starting with a dot character are interpreted as absolute imports.
- The sorted() function and the list's sort() method no longer accept the cmp argument. The key argument should be used instead.
- Division expressions on integers such as one half return floats. The truncating behavior is achieved through the // operator like 1//2. The good thing is that this can be used with floats too, so 5.0//2.0 == 2.0.