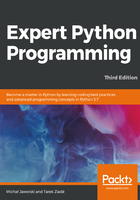
Dictionaries
Dictionaries are one of most versatile data structures in Python. The dict type allows you to map a set of unique keys to values, as follows:
{ 1: ' one', 2: ' two', 3: ' three', }
Dictionary literals are a very basic thing, and you should already know about them. Python allows programmers to also create a new dictionary using comprehensions, similar to the list comprehensions mentioned earlier. Here is a very simple example that maps numbers in a range from 0 to 99 to their squares:
squares = {number: number**2 for number in range(100)}
What is important is that the same benefits of using list comprehensions apply to dictionary comprehensions. So, in many cases, they are more efficient, shorter, and cleaner. For more complex code, when many if statements or function calls are required to create a dictionary, the simple for loop may be a better choice, especially if it improves readability.
For Python programmers new to Python 3, there is one important note about iterating over dictionary elements. The keys(), values(), and items() dictionary methods are no longer return lists. Also, their counterparts, iterkeys(), itervalues(), and iteritems(), which returned iterators instead, are missing in Python 3. Now, the keys(), values(), and items() methods return special view objects:
- keys(): This returns the dict_keys object which provides a view on all keys of the dictionary
- values(): This returns the dict_values object which provides a view on all values of the dictionary
- items(): This returns the dict_items object, providing views on all (key, value) two-tuples of the dictionary
View objects provide a view on the dictionary content in a dynamic way so that every time the dictionary changes, the views will reflect these changes, as shown in this example:
>>> person = {'name': 'John', 'last_name': 'Doe'}
>>> items = person.items()
>>> person['age'] = 42
>>> items
dict_items([('name', 'John'), ('last_name', 'Doe'), ('age', 42)])
View objects join the behavior of lists returned by the implementation of old methods with iterators that have been returned by their iter counterparts. Views do not need to redundantly store all values in memory (like lists do), but are still allowed to access their length (using the len() function) and testing for membership (using the in keyword). Views are, of course, iterable.
The last important thing about views is that both view objects returned by the keys() and values() methods ensure the same order of keys and values. In Python 2, you could not modify the dictionary content between these two calls if you wanted to ensure the same order of retrieved keys and values. dict_keys and dict_values are now dynamic, so even if the content of the dictionary changes between the keys() and values() calls, the order of iteration is consistent between these two views.