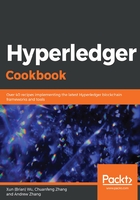
How it works...
A wallet can hold multiple identities. These identities are issued by the CA. As we have seen before, each user identity contains a certificate, X.509, which contains a private key and a public key, and some Fabric-specific metadata. The certificate file is issued from the Fabric CA service:

The blockchain admin creates a wallet to grant user access, then calls a couple of key class methods, (including X509WalletMixin.createIdentity) to manage the wallets and identities (including Org1MSP). This is shown in the following code:
const caURL = ccp.certificateAuthorities['ca.example.com'].url;
const ca = new FabricCAServices(caURL);
// Create a new file system based wallet for managing identities.
const walletPath = path.join(process.cwd(), 'wallet');
const wallet = new FileSystemWallet(walletPath);
..
// Enroll the admin user, and import the new identity into the wallet.
const enrollment = await ca.enroll({ enrollmentID: 'admin', enrollmentSecret: 'adminpw' });
const identity = X509WalletMixin.createIdentity('Org1MSP', enrollment.certificate,
enrollment.key.toBytes());
wallet.import('admin', identity);
X509WalletMixin.createIdentity is used to create an Org1MSP identity using X.509 credentials. The function needs three input: mspid, the certificate, and the private key.
From the connection.json file, we can see that the Org1MSP identity is associated with peer0.org1.example.com:
"organizations": {
"Org1": {
"mspid": "Org1MSP",
"peers": [
"peer0.org1.example.com"
],
"certificateAuthorities": [
"ca.example.com"
]
}
}
The gateway reads the connected profile, and the SDK will connect with the profile to manage the transaction submission and notification processes. In a basic-networkdocker-compose.yml file, the ca.example.com CA container starts fabric-ca-server to manage the Fabric CA key files:
ca.example.com:
image: hyperledger/fabric-ca
environment:
- FABRIC_CA_HOME=/etc/hyperledger/fabric-ca-server
- FABRIC_CA_SERVER_CA_NAME=ca.example.com
- FABRIC_CA_SERVER_CA_CERTFILE=/etc/hyperledger/fabric-ca-server-config/ca.org1.example.com-
cert.pem
- FABRIC_CA_SERVER_CA_KEYFILE=/etc/hyperledger/fabric-ca-server-config
/4239aa0dcd76daeeb8ba0cda701851d14504d31aad1b2ddddbac6a57365e497c_sk
ports:
- "7054:7054"
command: sh -c 'fabric-ca-server start -b admin:adminpw'
volumes:
- ./crypto-config/peerOrganizations/org1.example.com/ca/:/etc/hyperledger/fabric-ca-server-
config
container_name: ca.example.com
networks:
- basic
Our peer1.org1.com with MSPID Org1MSP is associated with the crypto-config file to verify each transaction:
peer0.org1.example.com:
container_name: peer0.org1.example.com
image: hyperledger/fabric-peer
environment:
- CORE_PEER_ID=peer0.org1.example.com
- CORE_PEER_LOCALMSPID=Org1MSP
- CORE_PEER_ADDRESS=peer0.org1.example.com:7051
volumes:
- ./crypto-config/peerOrganizations/org1.example.com/peers/peer0.org1.example.com/msp:/etc/hyperledger/msp/peer
- ./crypto-config/peerOrganizations/org1.example.com/users:/etc/hyperledger/msp/users
This will load the chaincode library and compile the Go code.