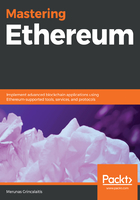
Strings and bytes
Strings and bytes hold pieces of text in single or double quotes, as follows:
string public myText = “This is a long text”;
bytes public myTextTwo = “This is another text”;
They allow you to store about 1,000 words and they are essentially the same. You can have smaller variations of bytes, such as bytes1, bytes2, and bytes3, up to bytes32.
Now, bytes32 is an interesting type of variable, because it allows you to store about 32 characters of text in a very compact and efficient way. They are used in many cases where short text is required:
bytes32 public shortText = “Short text.”;
They are used in many other advanced uses cases, such as checking if a string or byte's text is empty. For instance, if you have a function that receives text, you may want to make sure that the text is not empty. Here's how you'd do it:
function example(string memory myText) public {
require(bytes(myText)[0] != 0);
}
Don't worry about the technicalities of the function. If you don't know or remember them yet, to check if a string is empty, you must do the following:
require(bytes(yourString)[0] != 0);
This tells the contract to make sure that the first letter of the string is not empty. That's the right way to check for empty strings. We do the same thing with bytes, but without the conversion to bytes.
Use them whenever you need to add special characters to your strings in Ethereum.