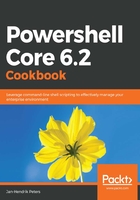
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's perform the following steps:
- As soon as you start PowerShell Core, the filesystem provider starts working and imports your mounted drives. You'll usually be placed in your own home directory or—as an administrator—in your system root.
- Have a look at the output of Get-PSProvider to see all available providers, as shown as follows:

- To see which cmdlets support providers, execute the following code:
# cmdlets that work with providers
Get-Command -Noun Location, Item, ItemProperty, ChildItem, Content, Path
- Different providers map different drives by default. Have a look at some of them by executing the following code:
# Providers usually automatically mount their drives
Get-PSDrive
- Providers allow you to use the same basic operations, such as Set-Location, on any path. Try it out with the filesystem:
# Navigate the filesystem
Set-Location -Path $home
- With the filesystem provider, the Get-ChildItem cmdlet can use additional parameters. Have a look at some of them with the next code sample:
# The File and FollowSymlink parameters are only available when the filesystem provider is used
Get-ChildItem -Recurse -File -FollowSymlink
Get-ChildItem -Path env: # Only default parameters here
# Globbing is supported regardless of the operating system and provider
Get-ChildItem -Path /etc/*ssh*/*config
Get-ChildItem -Path C:\Windows\*.dll
Get-ChildItem -Path env:\*module*
- It pays to have a look at the syntax of a cmdlet with Get-Command syntax first. Take a look at the following code block:
# Take a look at the syntax for easier operations
# e.g. Creating multiple items from an array
$folders = @(
"$home/test1"
"$home/test2/sub1/sub2"
"$home/test3"
)
New-Item -Path $folders -ItemType Directory -Force
# or creating a file in multiple locations
New-Item -Path $folders -Name 'someconfig.ini' -ItemType File -Value 'key = value'
- The filesystem provider behaves like the usual filesystem management tools of your operating system, but your results may vary when, for example, comparing the output of the Windows Explorer properties window with the number of files returned by Get-ChildItem:
New-Item $home\hidden\testfile,$home\hidden\.hiddentestfile -ItemType File -Force
$(Get-Item $home\hidden\.hiddentestfile -Force).Attributes = [System.IO.FileAttributes]::Hidden
Get-ChildItem -Path $home\hidden # .hiddentestfile won't appear
Get-ChildItem -Path $home\hidden -Hidden # Only shows the hidden file
Get-ChildItem -Path $home\hidden -Force # Retrieves all files
- The Include and Exclude parameters can be powerful filter parameters with Get-ChildItem:
# The Include and Exclude parameters can be useful filters
# / on Windows defaults to system drive
# Enables more complex filters than the Filter parameter
Get-ChildItem -Path $pshome -Recurse -Include *.dll,*.json -Exclude deps.ps1
- content cmdlets allow you to modify anything. With the filesystem, you would usually use them to read and modify file contents:
# This works for other Provider cmdlets as well
Get-Content -Path $pshome/Modules/PackageManagement/* -Include *.psm1
Set-Content -Path $home\testfile -Value "File content`nMultiple lines"
Add-Content -Path $home\testfile -Value 'Another new line'
Get-Content -Path $home\testfile
- Lastly, execute the following code to map a new CIFS share with PowerShell:
# The filesystem provider is one that allows mounting more provider drives
New-PSDrive -Name onlyInShell -Root \\someserver\someshare -PSProvider FileSystem
Get-ChildItem -Path onlyInShell:
Remove-PSDrive -Name onlyInShell