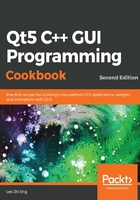
How it works...
The preceding example is a very simple application that showcases the use of lambda expressions to connect a signal with a lambda function or a regular function without declaring any slot function, and thus does not need to be inherited from a QObject class. This is especially useful for calling asynchronous processes that are not under UI objects.
Lambda expressions are functions that get defined within another function anonymously, which is quite similar to the anonymous functions in JavaScript. The format of a lambda function looks like this:
[captured variables](arguments) {
lambda code
}
You can insert variables into a lambda expression by placing them into the captured variables part, like we did in the example project in this recipe. We capture the QNetworkReply object called reply, and the QString object called html, and put them in our lambda expression.
Then, we can use these variables within our lambda code, as shown in the following code:
[reply, html]() {
html->append(QString(reply->readAll()));
}
The argument part is similar to an ordinary function, where you input values to the arguments and use them within your lambda code. In this case, the values of bytesReceived and bytesTotal are coming from the downloadProgress signal:
QObject::connect(reply, &QNetworkReply::downloadProgress,
[reply](qint64 bytesReceived, qint64 bytesTotal) {
qDebug() << "Progress: " << bytesReceived << "bytes /" << bytesTotal << "bytes";
});
You can also capture all variables that are used in your function using the equals sign. In this case, we captured the html variable without specifying it in the captured variable area:
[=]() {
printHTML(*html);
}