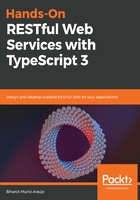
HTTP methods for RESTful services
The use of HTTP verbs allows a clear understanding of what an operation is going to do. In general, the primary or most commonly used HTTP verbs are POST, GET, PUT, PATCH, and DELETE, which stand for create, read, update (PATCH and PUT), and delete, respectively. Of course, there are also a lot of other verbs, but they are not used as frequently:

In order to explain these methods in detail, let's consider a simple entity called Customer. We will imagine that there is an API called Customers available through HTTP and its destination is a NoSQL database like in the following diagram:

Considering that the database is empty, what should happen if we call the GET method by pointing to GET /CUSTOMERS? If you think that it should retrieve nothing, then you are absolutely right! Here is a request example:
GET https://<HOST>/customers
And here is the response:
[]
So, considering that there is no customer yet, let's create one using the POST method:

Considering the POST method is successful, the HTTP status code should be 201 (we will talk more about status codes in Chapter 2, Principles of Designing RESTful APIs) and calling the GET method now; the response should be similar to the following code block (we're considering that there is just one single consumer of this endpoint and we are that consumer, so we can guarantee the data behavior into the server):

What if we add two more customers and call the GET method again? Take a look at the following code block:

Nice, so if we call the GET method for a specific ID, the response should be like the following:

We can also call POST methods for parent resources, such as /customers/1/orders, which will create new resources under the customer with ID 1. The same applies to the GET method, but only to retrieve the data as mentioned previously.
Okay, now that we know how to create and retrieve the resources, what if we want to change any information, such as the last name for John Doe? This one is easy—we just have to call the PATCH method in the same way that we called the POST method:

We can also change the information in a parent using a path as follows:

And what if we need to change the whole resource? In that case, we should use the PUT method:

Instead of PATCH, you can use the PUT method. To do so, all the parameters are required to be passed as the body into the request, even those parameters that haven't changed.
Finally, if you want to remove a resource, use the DELETE method:
