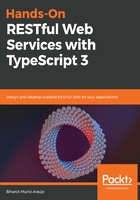
Pagination
Let's treat this issue like a real scenario. Considering that there is an endpoint that retrieves all orders from a retail company, such as GET /orders, the most obvious idea is to use a pagination strategy to allow the requester to define how many records they want to get. Thus, the requester has to send a query parameter telling the server how many items should be retrieved, given the limit and offset parameters. The offset and limit query parameters are very popular and are being adopted as a pattern for pagination. Basically, the offset will tell the service to start, based on the value passed as a parameter—the limit will tell you how long it will run for.
The following URI shows an example of calling an endpoint with pagination in place:
GET /items?offset=100&limit=20
This request will retrieve all items, starting from item number 100 until 120. At the moment, there is no sort strategy, so the result will be simply the first 20 items after 100:
[
{
resource_100
},
...
{
resource_119
}
]
There is also another possibility—using keyset or pagination links as the continuation point. It often uses timestamps as a key. For example, take the GET /items?limit=20 request. The keyset values are as follows:
- first: The first item based on the sort strategy chosen
- previous: The previous item based on the sort strategy chosen
- self: The item itself
- next: The next item based on the sort strategy chosen
- last: The last item based on the sort strategy chosen
The following example shows how to use paging information with the next and previous buckets:
{
"data" : [
{
resource 1
},
{
resource 2
},
...
{
resource 20
}
],
"paging": {
"previous": "https://{URI}/items?since=TIMESTAMP1"
"next": "https://{URI}/items?since=TIMESTAMP2"
}
}
We could also use the since query parameter based on the timestamp to get the previous and next batch of data based on the response of the first request. If we took the preceding response as an example and hit next, we'd get data starting on the last result on the first request we made, which means 21 and so on. Of course, we can combine the limit query parameter as well, for example, GET /items?since={TIMESTAMP}&limit=20.