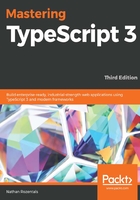
Classes
A class is a definition of an object, what data it holds, and what operations it can perform. Classes and interfaces form a cornerstone of the principles of object-oriented programming. Let's take a look at a simple class definition:
class SimpleClass { id: number; print() : void { console.log(`SimpleClass.print() called`); } }
Here, we have used the class keyword to define a class named SimpleClass, and we have defined this class to have a property named id, and a print function. The id property has been defined as type number. The print function simply logs a message to the console. Note that all we have done here is to define what data this class can hold, and what it can do. If we attempt to compile this code, however, we will generate an error, as follows:
error TS2564: Property 'id' has no initializer and is not definitely assigned in the constructor
What this error is indicating is that the id property, without being explicitly set, can in effect, still be undefined. If we attempt to use the id property, and it has not been set to a numeric value, then we might get a surprise if it is undefined. There are a number of ways to handle this error, but, for the time being, we can simply make the id property a type union, as follows:
id: number | undefined;
By adding the type union here, we are therefore making a conscious decision that we are going to allow the id property to possibly be undefined. This is an example of how the TypeScript compiler can pick up errors within our code that we may not have thought of initially.
In order to use this class definition, we need to create an instance of this class, which can be done as follows:
let mySimpleClass = new SimpleClass(); mySimpleClass.print();
Here, we define a variable named mySimpleClass to hold an instance of the SimpleClass class, and we then create this class using the new keyword. Once an instance of this class has been created, we can then call the print function on our class instance. The output of this code would be as follows:
SimpleClass.print() called