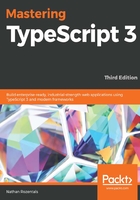
The any type
All this type checking is well and good, but JavaScript does not have strong typing, and allows variables to be mixed and matched. Consider the following code snippet that is actually valid JavaScript code:
var item1 = { id: 1, name: "item 1" }; item1 = { id: 2 };
Here, we assign an object with an id property and a name property to the variable item1. We then reassign this variable to an object that has an id property but not a name property. Unfortunately, as we have seen previously, this is not valid TypeScript code, and will generate the following error:
error TS2322: Type 'string[]' is not assignable to type 'number[]'.
TypeScript introduces the any type for such occasions. Specifying that an object has a type of any, in essence, relaxes the compiler's strict type checking. The following code shows how to use the any type:
var item1 : any = { id: 1, name: "item 1" }; item1 = { id: 2 };
Note how our first line of code has changed. We specify the type of the item1 variable to be of type : any. This special TypeScript keyword then allows a variable to follow JavaScript's loosely defined type rules, so that anything can be assigned to anything. Without the type specifier of : any, the second line of code would normally generate an error.