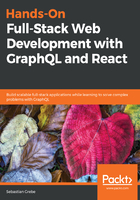
Setting up Express.js
As always, we need a root file loaded with all the main components that combines them to a real application.
Create an index.js file in the server folder. This file is the starting point for the back end. Here's how we go about it:
- First, we import express from node_modules, which we just installed. We can use import here since our back end gets transpiled by Babel. We are also going to set up webpack for the server-side code in a later in Chapter 9, Implementing Server-Side Rendering.
import express from 'express';
- We initialize the server with the express command. The result is stored in the app variable. Everything our back end does is executed through this object.
const app = express();
- Then, we specify the routes that accept requests. For this straightforward introduction, we accept all HTTP GET requests matching any path, by using the app.get method. Other HTTP Methods are catchable with app.post, app.put, and so on.
app.get('*', (req, res) => res.send('Hello World!'));
app.listen(8000, () => console.log('Listening on port 8000!'));
To match all paths, you use an asterisk, which generally stands for any in the programming environment, as we have done it in the preceding app.get line.
The first parameter for all app.METHOD functions are the path to match. From here, you can provide an unlimited list of callback functions, which are executed one by one. We are going to look at this feature later in the Routing with Express.js section.
A callback always receives the client request as the first parameter and the response as the second parameter, which the server is going to send. Our first callback is going to use the send response method.
The send function sends merely the HTTP response. It sets the HTTP body as specified. So, in our case, the body shows Hello World!, and the send function takes care of all necessary standard HTTP headers, such as Content-Length.
The last step to make our server publicly available is to tell Express.js on which port it should listen for requests. In our code, we are using 8000 as the first parameter of app.listen. You can replace 8000 with any port or URL you want to listen on. The callback is executed when the HTTP server binding has finished, and requests can be accepted on this port.
This is the easiest setup we can have for Express.js.