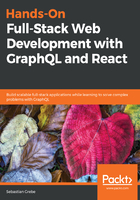
Routing in Express.js
Understanding routing is essential to extend our back end code. We are going to play through some simple routing examples.
In general, routing stands for how an application responds to specific endpoints and methods.
In Express.js, one path can respond to different HTTP methods and can have multiple handler functions. These handler functions are executed one by one in the order they were specified in the code. A path can be a simple string, but also a complex regular expression or pattern.
When using multiple handler functions—either provided as an array or multiple parameters—be sure to pass next to every callback function. When you call next, you hand over the execution from one callback function to the next function in the row. Those functions can also be middleware. We'll cover this in the next section.
Here is a simple example. Replace this with the current app.get line:
app.get('/', function (req, res, next) {
console.log('first function');
next();
}, function (req, res) {
console.log('second function');
res.send('Hello World!');
});
When you look at the server logs in the terminal, you will see both first function and second function printed. If you remove the execution of next and try to reload the browser tab, the request will time out. This problem occurs because neither res.send nor res.end, or any alternative is called. The second handler function is never executed when next is not run.
As previously discussed, the Hello World! message is nice but not the best we can get. In development, it is completely okay for us to run two separate servers: one for the front end and one for the back end.