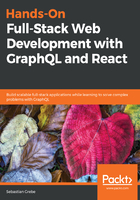
Express Helmet
Helmet is a tool that allows you to set various HTTP headers to secure your application.
We can enable the Express.js Helmet middleware as follows in the server index.js file:
app.use(helmet());
app.use(helmet.contentSecurityPolicy({
directives: {
defaultSrc: ["'self'"],
scriptSrc: ["'self'", "'unsafe-inline'"],
styleSrc: ["'self'", "'unsafe-inline'"],
imgSrc: ["'self'", "data:", "*.amazonaws.com"]
}
}));
app.use(helmet.referrerPolicy({ policy: 'same-origin' }));
We are doing multiple things here at once. We add some XSS(Cross-Site-Scripting) protection tactics and remove the X-Powered-By HTTP header and some other useful things just by using the helmet() function in the first line.
Furthermore, to ensure that no one can inject malicious code, we are using the Content-Security-Policy HTTP header or, in short, CSP. This header prevents attackers from loading resources from external URLs.
As you can see, we also specify the imgSrc field, which tells our client that only images from these URLs should be loaded, including Amazon Web Services (AWS). We will see how to upload images to it in Chapter 7, Handling Image Uploads, of this book.
Read more about CSP and how it can make your platform more secure at, https://helmetjs.github.io/docs/csp/.
The last enhancement is to set the Referrer HTTP header only when making requests on the same host. When going from domain A to domain B, for example, we do not include the referrer, which is the URL the user is coming from. This enhancement stops any internal routing or requests being exposed to the internet.
It is important to initialize Helmet very high in your Express router so that all responses are affected.