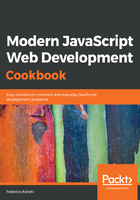
Adding Flow for data types checks
Let's finish this chapter by considering a tool that turns JS into a (sort of) new language, a typed one. One of the characteristics of JS is being untyped; for example, a variable can hold, or a function may return, any kind of value, there's no way to declare what type(s) should be stored in a variable or returned from a function. In this section, we will add Flow, a tool developed by Facebook, which allows for data type controls.
We will be getting into how to fully use Flow in the Adding types section of Chapter 2, Using JavaScript Modern Features, but let me give you a preview of what we expect; then, we'll get to install all the needed packages, and afterwards we'll go into more details. Imagine you wrote a highly complex function to add two numbers:
function addTwoNumbers(x, y) {
return x + y;
}
console.log(addTwoNumbers(22, 9)); // 31, fine
However, since JS won't check types and has some conversion rules, the following two lines would also work:
console.log(addTwoNumbers("F", "K")); // FK - oops...
console.log(addTwoNumbers([], {})); // [object Object]! more oops...
You could, on principle, add a lot of data type checking code to your function to verify typeof(x)==="number", but that can become a chore. (Although, of course, for some cases it's the only solution.) However, many errors can be detected before even running the code, as would happen here.
If you modify the function to include data type declarations, Flow will be able to detect the two wrong uses, and you will be able to solve the situation before even running the code:
function addTwoNumbers(x: number, y: number) {
return x + y;
}
Basically, that's all there is! Of course, there are many details about what data types are available, defining new ones, using interfaces, and much more, but we'll get to that in the next chapter. For the time being, let's just install it with the promise that we will learn more about its use very shortly.