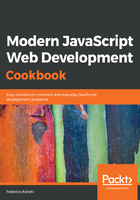
Generic types
In functional programming, it's quite usual to work with the identity function, which is defined as follows:
// Source file: src/types_advanced.js
const identity = x => x;
How would you write a type definition for this function? If the argument is a number, it will return a number; if it's a string, it'll return a string and so on. Writing all possible situations would be a chore and not very Don't Repeat Yourself (DRY). Flow provides a solution, with generic types:
// Source file: src/types_advanced.js
const identity = <T>(x: T): T => x;
In this case, T stands for the generic type. Both the argument of the function and the result of the function itself are defined to be of T type, so Flow will know that whatever type the argument is, the result type will be the same. A similar syntax would be used for the more usual way of defining functions:
// Source file: src/types_advanced.js
function identity2<T>(x: T): T {
return x;
}
Flow also checks that you don't accidentally restrict a generic type. In the following case, you would always be returning a number, while T might actually be any other different type:
// Source file: src/types_advanced.js
function identity3<T>(x: T): T {
return 229; // wrong; this is always a number, not generic
}
You need not restrict yourself to a single generic type; the following nonsense example shows a case with two types:
// Source file: src/types_advanced.js
function makeObject<T1, T2>(x: T1, y: T2) {
return { first: x, second: y };
}
It's also possible to define a parametric type with a generic type that can later be specified. In the following example, the type definition for pair allows you to further create new types, each of which will always produce pairs of values of the same type:
// Source file: src/types_advanced.js
type pair<T> = [T, T];
type pairOfNumbers = pair<number>;
type pairOfStrings = pair<string>;
let pn: pairOfNumbers = [22, 9];
let ps: pairOfStrings = ["F", "K"];