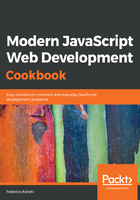
Working with libraries
Today, it's highly likely that any project you create will depend on third-party libraries, and it's very likely that those weren't written with Flow. By default, Flow will ignore these libraries and won't do any type checking. This means that any data type errors you might commit when using the library will be unrecognized, and you'll have to deal with them in the old-fashioned way, through testing and debugging—a throwback to worse times!
To solve this problem, Flow lets you work with library definitions (libdefs) (see https://flow.org/en/docs/libdefs/) that describe the data types, interfaces, or classes for a library, separately from the library itself, like header files in C++ and other languages. Libdefs are .js files, but they are placed in a flow-typed directory at the root of your project.
There exists a repository of library definitions, flow-typed, in which you can find already made files for many popular libraries; see https://github.com/flowtype/flow-typed for more information. However, you don't need to directly deal with that, because there is a tool that does the work for you, though at some times it will pass the buck back to you!
First, install the new tool:
npm install flow-typed --save-dev
Then, add a script in package.json to simplify the work:
scripts: {
.
.
.
addTypes: "flow-typed install",
.
.
.
Using npm run addTypes will scan your project and attempt to add all possible libdefs. If it cannot find an appropriate definition for a library (this isn't unusual, I'm sorry to say), it will create a basic definition using any everywhere. For instance, I added the moment library to the project:
> npm install moment --save
> npm run addTypes
After this, the flow-typed directory was added to the project root. In it, there a lot of files appeared, including moment_v2.3.x.js with the type definitions for the moment library. For libraries without a libdef, files were also created, but you may ignore them.
If you need a libdef, and it doesn't exist, you may be able to create it by yourself. (And, please, contribute your work to the flow-typed project!) I added npm install fetch --save, but when I tried to get the libdef, it wasn't found. So, I can either keep working without the definitions (the standard situation!) or I can try to create the appropriate file; none is really an optimal situation.