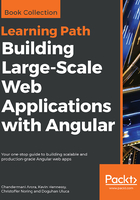
Property binding continued...
Now that we understand the difference between a property and an attribute, let's look at the binding example again:
<img class="img-responsive" [src]="'/static/images/' + currentExercise.exercise.image" />
The [propertName] square bracket syntax is used to bind the img.src property to an Angular expression.
The general syntax for property binding looks as follows:
[target]="sourceExpression";
In the case of property binding, the target is a property on the DOM element or component. With property binding, we can literally bind to any property on the element's DOM. The src property on the img element is what we use; this binding works for any HTML element and every property on it.
It is important to understand the difference between source and target in an Angular binding. The property appearing inside [] is a target, sometimes called binding target. The target is the consumer of the data and always refers to a property on the component/element. The source expression constitutes the data source that provides data to the target.
At runtime, the expression is evaluated in the context of the component's/element's property (the WorkoutRunnerComponent.currentExercise.exercise.image property in the preceding case).
Property binding, event binding, and attribute binding do not use the interpolation symbol. The following is invalid: [src]="{{'/static/images/' + currentExercise.exercise.image}}".
From a data binding perspective, Angular treats components in the same way as it treats native elements. Hence, property binding works on component properties too! Components can define input and output properties that can be bound to the view, such as this:
<workout-runner [exerciseRestDuration]="restDuration"></workout-runner>
This hypothetical snippet binds the exerciseRestDuration property on the WorkoutRunnerComponent class to the restDuration property defined on the container component (parent), allowing us to pass the rest duration as a parameter to the WorkoutRunnerComponent. As we enhance our app and develop new components, you will learn how to define custom properties and events on a component.
The template view that we just created has only one property binding (on [src]). The other bindings with square brackets aren't property bindings. We will cover them shortly.