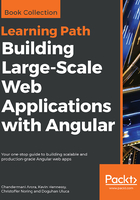
Angular pipes
The primary aim of a pipe is to format the data displayed in the view. Pipes allow us to package this content transformation logic (formatting) as a reusable element. The framework itself comes with multiple predefined pipes, such as date, currency, lowercase, uppercase, slice, and others.
This is how we use a pipe with a view:
{{expression | pipeName:inputParam1}}
An expression is followed by the pipe symbol (|), which is followed by the pipe name and then an optional parameter (inputParam1) separated by a colon (:). If the pipe takes multiple inputs, they can be placed one after another separated by a colon, such as the inbuilt slice pipe, which can slice an array or string:
{{fullName | slice:0:20}} //renders first 20 characters
The parameter passed to the pipe can be a constant or a component property, which implies we can use template expressions with pipe parameter. See the following example:
{{fullName | slice:0:truncateAt}} //renders based on value truncateAt
Here are some examples of the use of the date pipe, as described in the Angular date documentation. Assume that dateObj is initialized to June 15, 2015 21:43:11 and locale is en-US:
{{ dateObj | date }} // output is 'Jun 15, 2015 ' {{ dateObj | date:'medium' }} // output is 'Jun 15, 2015, 9:43:11 PM' {{ dateObj | date:'shortTime' }} // output is '9:43 PM ' {{ dateObj | date:'mmss' }} // output is '43:11'
Some of the most commonly used pipes are the following:
- date: As we just saw, the date filter is used to format the date in a specific manner. This filter supports quite a number of formats and is locale-aware too. To know about the other formats supported by the date pipe, check out the framework documentation at http://bit.ly/ng2-date.
- uppercase and lowercase: These two pipes, as the name suggests, change the case of the string input.
- decimal and percent: decimal and percent pipes are there to format decimal and percentage values based on the current browser locale.
- currency: This is used to format numeric values as a currency based on the current browser locale:
{{14.22|currency:"USD" }} <!-Renders USD 14.22 --> {{14.22|currency:"USD":'symbol'}} <!-Renders $14.22 -->
- json: This is a handy pipe for debugging that can transform any input into a string using JSON.stringify. We made good use of it at the start of this chapter to render the WorkoutPlan object (see the Checkpoint 2.1 code).
- slice: This pipe allows us to split a list or a string value to create a smaller trimmed down list/string. We saw an example in the preceding code.
We are not going to cover the preceding pipes in detail. From a development perspective, as long as we know what pipes are there and what they are useful for, we can always refer to the platform documentation for exact usage instructions.