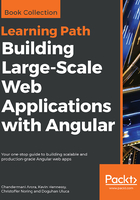
Getting started with Personal Trainer navigation
At this point, if you look at the route configuration in app-routing.module.ts in the src/app folder, you will find one new route definition, builder:
const routes: Routes = [
...
{ path: 'builder', component: WorkoutBuilderComponent },
...
];
And if you run the application, you will see that the start screen shows another link, Create a Workout:

Behind the scenes, we have added another router link for this link into start.component.html:
<a routerLink="/builder" class="btn btn-primary btn-lg btn-block" role="button" aria-pressed="true">
<span>Create a Workout</span>
<span class="ion-md-add"></span>
</a>
And if you click on this link, you will be taken to the following view:

Again, behind the scenes we have added workout-builder.component.ts to the trainer/src/app/workout-builder folder with the following inline template:
template: `
<div class="row">
<div class="col-sm-3"></div>
<div class="col-sm-6">
<h1 class="text-center">Workout Builder</h1>
</div>
<div class="col-sm-3"></div>
</div>
`
And this view is displayed on the screen under the header using the router outlet in our app.component.html template:
<div class="container body-content app-container"> <router-outlet></router-outlet> </div>`
We have wrapped this component (along with the other files we have stubbed out for this feature) in a new module named workout-builder.module.ts:
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { WorkoutBuilderComponent } from './workout-builder.component';
import { ExerciseComponent } from './exercise/exercise.component';
import { ExercisesComponent } from './exercises/exercises.component';
import { WorkoutComponent } from './workout/workout.component';
import { WorkoutsComponent } from './workouts/workouts.component';
@NgModule({
imports: [
CommonModule
],
declarations: [WorkoutBuilderComponent, ExerciseComponent, ExercisesComponent, WorkoutComponent, WorkoutsComponent]
})
export class WorkoutBuilderModule { }
The only thing that might look different here from the other modules that we have created is that we are importing CommonModule instead of BrowserModule. This avoids importing the whole of BrowserModule a second time, which would generate an error when we get to implementing lazy loading for this module.
Finally, we have added an import for this module to app.module.ts:
... @NgModule({ imports: [ ... WorkoutBuilderModule], ...
So, nothing surprising here. Following these patterns, we should now begin to think about adding the additional navigation outlined previously for our new feature. However, before we jump into doing that, there are a couple of things we need to consider.
First, if we start adding our routes to the app.routing-module.ts file, then the number of routes stored there will grow. These new routes for Workout Builder will also be intermixed with the routes for Workout Runner. While the number of routes we are now adding might seem insignificant, over time this could get to be a maintenance problem.
Second, we need to take into consideration that our application now consists of two features—Workout Runner and Workout Builder. We should be thinking about ways to separate these features within our application so that they can be developed independently of each other.
Put differently, we want loose coupling between the features that we build. Using this pattern allows us to swap out a feature within our application without affecting the other features. For example, somewhere down the line, we may want to convert the Workout Runner into a mobile app but leave the Workout Builder intact as a web-based application.
This ability to separate our components from each other is one of the key advantages of using the component design pattern that Angular implements. Fortunately, Angular's router gives us the ability to separate out our routing into logically organized routing configurations that closely match the features in our application.
In order to accomplish this separation, Angular allows us to use child routing, where we can isolate the routing for each of our features. In this chapter, we will use child routing to separate out the routing for Workout Builder.