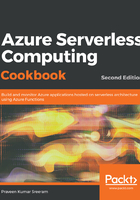
上QQ阅读APP看书,第一时间看更新
How to do it...
Perform the following steps:
- Navigate to your function app, create a new HTTP trigger using the HttpTrigger-CSharp template, and choose Anonymous for the Authorization level.
- Navigate to the code editor of run.csx in the SaveJSONToAzureSQLDatabase function and replace the default code with the following. The following code grabs the data that is passed to the HTTP trigger and inserts it into the database using the ADO.Net API:
#r "Newtonsoft.Json"
#r "System.Data"
using System.Net;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Primitives;
using Newtonsoft.Json;
using System.Data.SqlClient;
using System.Data;
public static async Task<IActionResult> Run(HttpRequest req, ILogger log)
{
log.LogInformation("C# HTTP trigger function processed a request.");
string firstname,lastname, email, devicelist;
string requestBody = await new StreamReader(req.Body).ReadToEndAsync();
dynamic data = JsonConvert.DeserializeObject(requestBody);
firstname = data.firstname;
lastname = data.lastname;
email = data.email;
devicelist = data.devicelist;
SqlConnection con =null;
try
{
string query = "INSERT INTO EmployeeInfo (firstname,lastname, email, devicelist) " + "VALUES (@firstname,@lastname, @email, @devicelist) ";
con = new
SqlConnection("Server=tcp:azurecookbooks.database.windows.net,1433;Initial Catalog=Cookbookdatabase;Persist Security Info=False;User ID=username;Password=password;MultipleActiveResultSets=False;Encrypt=True;TrustServerCertificate=False;Connection Timeout=30;");
SqlCommand cmd = new SqlCommand(query, con);
cmd.Parameters.Add("@firstname", SqlDbType.VarChar,
50).Value = firstname;
cmd.Parameters.Add("@lastname", SqlDbType.VarChar, 50)
.Value = lastname;
cmd.Parameters.Add("@email", SqlDbType.VarChar, 50)
.Value = email;
cmd.Parameters.Add("@devicelist", SqlDbType.VarChar)
.Value = devicelist;
con.Open();
cmd.ExecuteNonQuery();
}
catch(Exception ex)
{
log.LogInformation(ex.Message);
}
finally
{
if(con!=null)
{
con.Close();
}
}
return (ActionResult)new OkObjectResult($"Successfully inserted the data.");
}
Note that you need to validate each and every input parameter. For the sake of simplicity, the code that validates the input parameters is not included. Make sure that you validate each and every parameter before you save it into your database. It's also good practice to store the connection string in Application settings.
- Let's run the HTTP trigger using the following test data, right from the Test console of Azure Functions:
{
"firstname": "Praveen",
"lastname": "Kumar",
"email": "praveen@example.com",
"devicelist":
"[
{
'Type' : 'Mobile Phone',
'Company':'Microsoft'
},
{
'Type' : 'Laptop',
'Company':'Lenovo'
}
]"
}
Note that you need to validate each and every input parameter. For the sake of simplicity, the code that validates the input parameters is not included. Make sure that you validate each and every parameter before you save it into your database.
A record was inserted successfully, as shown in the following screenshot: