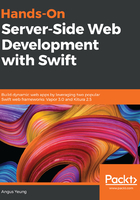
上QQ阅读APP看书,第一时间看更新
Creating a new entry with a unique ID
Following getTotal(), add the following handler for creating a new entry:
func newEntry(_ req: Request) throws -> Future<HTTPStatus> { // [1]
let newID = UUID().uuidString // [2]
return try req.content.decode(Entry.self).map(to: HTTPStatus.self) { entry in // [3]
let newEntry = Entry(id: newID,
title: entry.title,
content: entry.content) // [4]
guard let result = self.journal.create(newEntry) else { // [5]
throw Abort(.badRequest) // [6]
}
print("Created: \(result)")
return .ok // [7]
}
}
The newEntry(_ req:) function handles the creation of a new entry in several steps:
- Constructs a throwable function that returns a Future of HTTPStatus
- Uses Swift's UUID() to create a unique ID for the new entry
- Decodes the JSON object embedded in an HTTP request and serializes to an entry instance
- Instantiates a new entry object using the new ID
- Invokes the create() function of RouteController to add the new entry object to in-memory storage
- Throws an Abort if the last step returns nil
- Returns HTTPStatus.ok when reaching the end of the function
In [4], whatever ID that has been submitted is simply ignored, since the id field is always over-written with a new UUID. This guarantees that the id is unique, and this is very useful for you to debug the CRUD operations you've implemented.
The Abort in [6] is a default implementation of the AboutError protocol. Vapor always displays any error code specified in Abort to the end user, even in production mode where most error messages are suppressed.