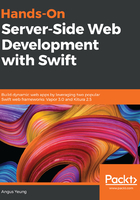
上QQ阅读APP看书,第一时间看更新
Adding more routes in Kitura
You can add more routes to your project as follows:
- Open Application.swift in Xcode and add the following code to the line right after initializeHealthRoutes(app: self) in the PostInit() function:
. . .
// add after initializeHealthRoutes(app: self)
router.get("/greet") { request, response, next in // [1]
if let guest = request.queryParameters["guest"] { // [2]
response.send("Hi \(guest), greetings! Thanks for visiting us.") // [3]
} else {
response.send("Hi stranger, It's nice meeting with you.")
}
}
router.get("/student/:name") { request, response, next in // [4]
let studentName = request.parameters["name"]!
let studentRecords = [ // [5]
"Peter" : 3.42,
"Thomas" : 2.98,
"Jane" : 3.91,
"Ryan" : 4.00,
"Kyle" : 4.00
]
var : String
if let gpa = studentRecords[studentName] {
queryResponse = "The student \(studentName)'s GPA is \(gpa)" // [6]
} else {
queryResponse = "The student's record can't be found!"
}
response.send(queryResponse) // [7]
. . .
- Similar to what you have done in the helloWorld Vapor project, the preceding code adds two routes, greet and student, to your Kitura project and processes them in the following ways:
- [1] Add the path greet with the query parameter guest
- [2] Retrieve the guest parameter from the request using queryParameters()
- [3] Send a response using response.send()
- [4] Add the path student followed by the path parameter's name
- [5] Create a dictionary with a name and GPA key-value pairs
- [6] Use the name as a key to retrieve the GPA and customize the response
- [7] Send the response with a customized message using response.send()
The first endpoint handles a request with query parameter and returns a response like this:
$ curl "http://localhost:8080/greet?guest=Neil"
Hi Neil, greetings! Thanks for visiting us. Unfortunately, there is nothing too much to see here.
The second endpoint handles a request with path parameter and returns the following response:
$ curl "http://localhost:8080/student/Jane"
The student Jane's GPA is 3.91
There are three parameters that are passed to a router's closure: request, response, and next in.
- The request parameter contains all the information about the incoming HTTP request. The /greet endpoint demonstrates a path with query parameters, that is, localhost:8080/greet?guest=Neil, you can use the request's queryParameter() to retrieve it. Kitura also allows you to use a path with a parameter, as shown in the /student endpoint. A path parameter is the parameter name prefixed with a colon. In the preceding code, you use the request object's parameters array to retrieve the path parameter. If you want, you can add as many path parameters as you want, as long as each path parameter is unique.
- The response parameter provides you with a flexible way to return data going out to the requestor. In this example, your response is simply a string message. However, you can also include status code, formatted headers, [String: Any] dictionary, or even a JSON object in your response.
- The next parameter is the next handler that should be invoked for the route. Kitura allows more than one handler for each endpoint. To let the next handler have an opportunity to run its code, you need to call next() right before you have finished using your router code:
. . .
router.get("/greet") { request, response, next in
if let guest = request.queryParameters["guest"] {
response.send("Hi \(guest), greetings! Thanks for visiting us.")
} else {
response.send("Hi stranger, It's nice meeting with you.")
}
next() // [1]
}
router.get("/greet") { request, response, next in
response.send(" Unfortunately, there is nothing too much to see here.")
}
. . .
In the preceding code, there are two handlers for the /greet endpoint. The next() call at [1] is added to the first handler of the /greet endpoint so the second handler of the /greet endpoint has a chance to handle the request. The output will look like this:
Try removing the next() statement and you will see that the second handler no longer works.