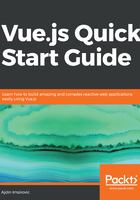
Mustache template example
Let's begin working with our first pen:
<div id="entryPoint">
<h1>Just an h1 heading here</h1>
<h2>Just an h2 heading here</h2>
<p>Vue JS is fun</p>
</div>
We can now see our HTML being rendered in the CodePen preview pane, with the following text printed on the screen:
Just an h1 heading here
Just an h2 heading here
Vue JS is fun
Next, let's add the following Vue code to the JS pane inside our pen:
new Vue({
el: '#entryPoint',
data: {
heading1: 'Just an h1 heading here',
heading2: 'heading 2 here',
paragraph1: 'Vue JS'
}
})
Finally, let's update the HTML so that the Vue code can work its magic:
<div id="entryPoint">
<h1>{{ heading1 }}</h1>
<h2>Just an {{ heading2 }}</h2>
<p>{{paragraph1}} is fun</p>
</div>
In the previous code example, we can see how we use mustache templates to dynamically insert data into our HTML.
As mentioned before, CodePen will auto-update the preview pane, but this will not affect the preview since we are effectively producing the same output as we did when we were using just plain HTML.
Now we can play with it simply by changing the key-value pairs inside our data entry:
new Vue({
el: '#entryPoint',
data: {
heading1: 'This is an h1',
heading2: 'h2 heading',
paragraph1: 'Vue2'
}
})
This time, the output will auto-update to this:
This is an h1
Just an h2 heading
Vue2 is fun
We can also change our entry point. For example, we can have Vue access only the p tag:
new Vue({
el: 'p',
data: {
heading1: 'This is an h1',
//heading2: 'h2 heading',
paragraph1: 'Vue2'
}
})
After this change, our preview pane will show the following:
{{ heading1 }}
Just an {{ heading2 }}
Vue2 is fun
From this output, we can conclude that our mustache templates will be rendered in our HTML output as regular text if either of the following things happen:
- Our entry point does not reference the data
- The entry in our data does not exist
We've also seen how our entry point can be any kind of selector. You can think of it as being similar to how you can target different elements in jQuery.
For example, we could have a more complex selector as our app's entry point:
new Vue({
el: 'div#entryPoint',
data: {
heading1: 'This is an h1',
heading2: 'h2 heading',
paragraph1: 'Vue2'
}
})