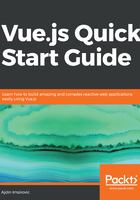
Understanding components, templates, and props
To begin, let's look at how to make a component in Vue. First, we specify the component, like this:
Vue.component('custom-article', {
template: `
<article>
Our own custom article component!<span></span>
</article>`
})
new Vue({
el: '#app'
})
A component is a block of code that we give a custom name. This custom name can be anything we come up with, and it's a single label for that entire block of code in the form of a custom HTML tag. In the previous example, we grouped the article and span tags and gave that custom tag the name of custom-article.
The code for this component is available as a Codepen at https://codepen.io/AjdinImsirovic/pen/xzpOaJ.
Now, to create an instance of our component, we simply use our <custom-article> opening and closing tags in our HTML, like this:
<main id="app">
<custom-article></custom-article>
</main>
The parent is the actual Vue instance.
Note that you can use string templates even without a component. You simply add the template option to your Vue instance, like this:
//HTML
<main id="app"></main>
//JS
new Vue({
el: '#app',
template: '<article>A string template without a component!<span></span></article>'
})
The example code for the previous example is available here: https://codepen.io/AjdinImsirovic/pen/RJxMae.
Next, we'll see how we can improve our component with the help of the props and data options.